In this tutorial we are going to see how to download file from server using Servlet.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
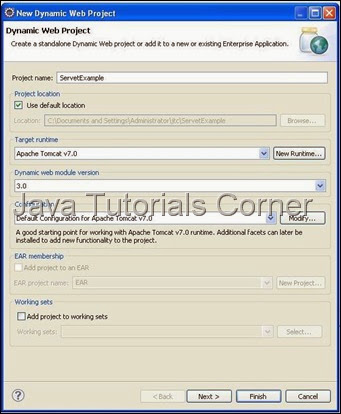
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called DownloadFileServlet as shown in figure.
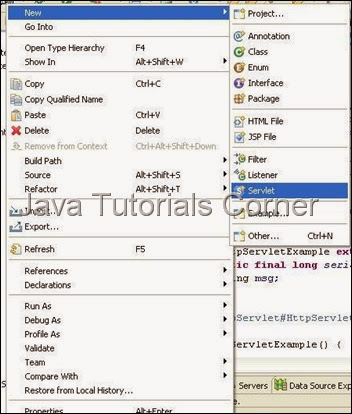
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add commons-io-1.3.2.jar in your build path, which used to convert InputStream to byte[]
9. Add the required code inside doGet() method.
DownloadFileServlet,java
In above program response.setContentType("image/jpeg"); used to specify the MIME type of file tobe downloaded.
response.setHeader("Content-Disposition","attachment; filename=\"Water lilies.jpg\""); Which ask user to save the file in disk with given name.
10. Create html page in WebContent folder
File-download.html
11. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
12.call the following URL.
http://localhost:8080/ServletExample/File-download.html
Output
Click Download Link
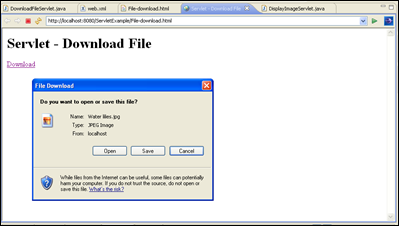
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
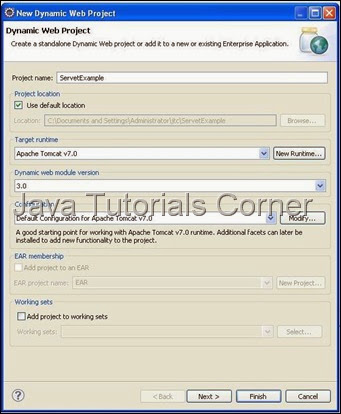
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called DownloadFileServlet as shown in figure.
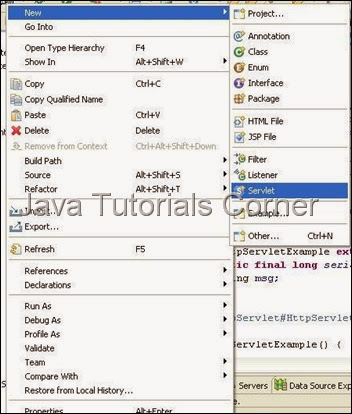
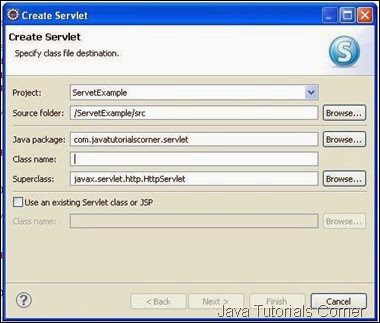
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
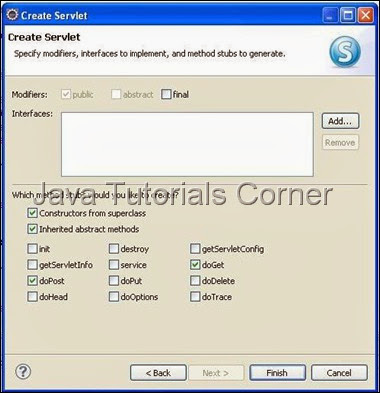
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>DownloadFileServlet</servlet-name> <servlet-class>com.javatutorialscorner.servlet.DownloadFileServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>DownloadFileServlet</servlet-name> <url-pattern>/DownloadFileServlet</url-pattern> </servlet-mapping> </web-app>
8. Add commons-io-1.3.2.jar in your build path, which used to convert InputStream to byte[]
9. Add the required code inside doGet() method.
DownloadFileServlet,java
package com.javatutorialscorner.servlet; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.commons.io.IOUtils; /** * Servlet implementation class DownloadFileServlet */ public class DownloadFileServlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public DownloadFileServlet() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub try { // you can use FileInputStream to convert any type of file to // InputStream InputStream inputStream = new FileInputStream( "C://jtc//Water lilies.jpg"); // IOUtils jar used to convert Input Stream to byte array easily byte[] bytes = IOUtils.toByteArray(inputStream); response.setContentType("image/jpeg"); response.setHeader("Content-Disposition","attachment; filename=\"Water lilies.jpg\""); OutputStream outputStream = response.getOutputStream(); outputStream.write(bytes); outputStream.close(); } catch (Exception e) { // TODO: handle exception e.printStackTrace(); } } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
In above program response.setContentType("image/jpeg"); used to specify the MIME type of file tobe downloaded.
response.setHeader("Content-Disposition","attachment; filename=\"Water lilies.jpg\""); Which ask user to save the file in disk with given name.
10. Create html page in WebContent folder
File-download.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Servlet - Download File</title> </head> <body> <h1>Servlet - Download File</h1> <a href="http://localhost:8080/ServletExample/DownloadFileServlet">Download</a> </body> </html>
11. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
12.call the following URL.
http://localhost:8080/ServletExample/File-download.html
Output

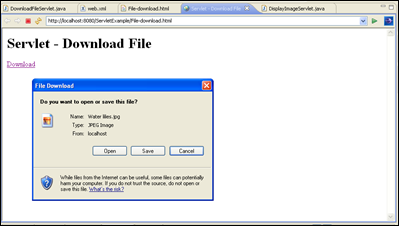
0 comments:
Post a Comment