In this tutorial we are going to see about HttpServlet with example program.
A Servlet is just normal class which implements the Interface Servlet.
The following classes implements the Servlet Interface.
You can create HTTP servlet by extends javax.servlet.http.HttpServlet . The javax.servlet.http.HttpServlet is an abstract class.
A subclass of HttpServlet must override at least one method, usually one of these:
1. doGet, if the servlet supports HTTP GET requests
2. doPost, for HTTP POST requests
3. doPut, for HTTP PUT requests
4. doDelete, for HTTP DELETE requests
5. init and destroy, to manage resources that are held for the life of the servlet
6. getServletInfo, which the servlet uses to provide information about itself
There's almost no reason to override the service method. service handles standard HTTP requests by dispatching them to the handler methods for each HTTP request type (the doXXX methods listed above).
Likewise, there's almost no reason to override the doOptions and doTrace methods.
Servlets typically run on multithreaded servers, so be aware that a servlet must handle concurrent requests and be careful to synchronize access to shared resources. Shared resources include in-memory data such as instance or class variables and external objects such as files, database connections, and network connections.
Now see how to create HttpServlet.Step by step procedure given below.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called HttpServletExample as shown in figure.
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
8. Add the required code inside service() method.
HttpServletExample.java
In above servlet program overrides service() method so all the request goes to service() method first.There's almost no reason to override the service method.
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
10.call the URL which is mapped in web.xml.
Output
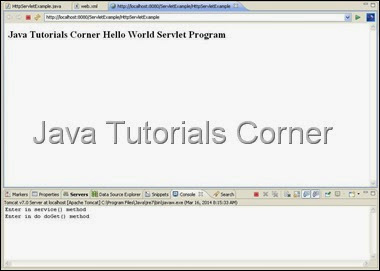
A Servlet is just normal class which implements the Interface Servlet.
javax.servlet.Servlet
The following classes implements the Servlet Interface.
import javax.servlet.GenericServlet;
import javax.servlet.http.HttpServlet;
You can create HTTP servlet by extends javax.servlet.http.HttpServlet . The javax.servlet.http.HttpServlet is an abstract class.
A subclass of HttpServlet must override at least one method, usually one of these:
1. doGet, if the servlet supports HTTP GET requests
2. doPost, for HTTP POST requests
3. doPut, for HTTP PUT requests
4. doDelete, for HTTP DELETE requests
5. init and destroy, to manage resources that are held for the life of the servlet
6. getServletInfo, which the servlet uses to provide information about itself
There's almost no reason to override the service method. service handles standard HTTP requests by dispatching them to the handler methods for each HTTP request type (the doXXX methods listed above).
Likewise, there's almost no reason to override the doOptions and doTrace methods.
Servlets typically run on multithreaded servers, so be aware that a servlet must handle concurrent requests and be careful to synchronize access to shared resources. Shared resources include in-memory data such as instance or class variables and external objects such as files, database connections, and network connections.
Now see how to create HttpServlet.Step by step procedure given below.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
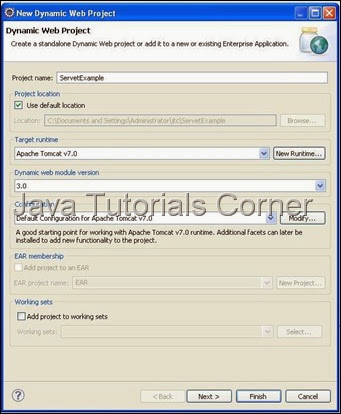
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called HttpServletExample as shown in figure.


5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet>web.xml
<servlet-name>Your Servlet Name</servlet-name>
<servlet-class>Fully Qulaified Servlet Class Name</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Your Servlet Name</servlet-name>
<url-pattern>/URL to Call Servlet</url-pattern>
</servlet-mapping>
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>ServetExample</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>HttpServletExample</servlet-name>
<servlet-class>com.javatutorialscorner.servlet.HttpServletExample</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HttpServletExample</servlet-name>
<url-pattern>/HttpServletExample</url-pattern>
</servlet-mapping>
</web-app>
8. Add the required code inside service() method.
HttpServletExample.java
package com.javatutorialscorner.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class HttpServletExample
*/
public class HttpServletExample extends HttpServlet {
private static final long serialVersionUID = 1L;
private String msg;
/**
* @see HttpServlet#HttpServlet()
*/
public HttpServletExample() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see Servlet#init(ServletConfig)
*/
public void init(ServletConfig config) throws ServletException {
// TODO Auto-generated method stub
msg = "Java Tutorials Corner Hello World Servlet Program";
}
/**
* @see Servlet#destroy()
*/
public void destroy() {
// TODO Auto-generated method stub
}
/**
* @see Servlet#getServletConfig()
*/
public ServletConfig getServletConfig() {
// TODO Auto-generated method stub
return null;
}
/**
* @see Servlet#getServletInfo()
*/
public String getServletInfo() {
// TODO Auto-generated method stub
return null;
}
/**
* @see HttpServlet#service(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void service(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
System.out.println("Enter in service() method");
doGet(request,response);
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
System.out.println("Enter in do doGet() method");
response.setContentType("text/html");
PrintWriter writer = response.getWriter();
writer.write("<html><body><h2>" + msg + "</h2></body></html>");
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
/**
* @see HttpServlet#doPut(HttpServletRequest, HttpServletResponse)
*/
protected void doPut(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
/**
* @see HttpServlet#doDelete(HttpServletRequest, HttpServletResponse)
*/
protected void doDelete(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
/**
* @see HttpServlet#doHead(HttpServletRequest, HttpServletResponse)
*/
protected void doHead(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
/**
* @see HttpServlet#doOptions(HttpServletRequest, HttpServletResponse)
*/
protected void doOptions(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
/**
* @see HttpServlet#doTrace(HttpServletRequest, HttpServletResponse)
*/
protected void doTrace(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
}
}
In above servlet program overrides service() method so all the request goes to service() method first.There's almost no reason to override the service method.
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
10.call the URL which is mapped in web.xml.
Output
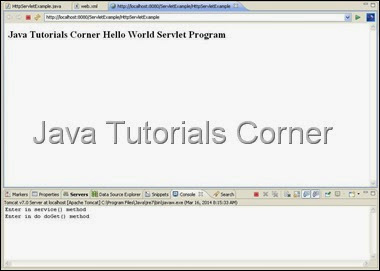
0 comments:
Post a Comment