In this tutorial we are going to see about, how to read Context param using ServletContext interface. In previous tutorials I explained about ServletContext interface with example program (read single context-param).Click here to read more about Servlet Context Interface.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called ServletContextExample as shown in figure.
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add the required code inside doGet() method.
ServletContextExample.java
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/ServletContextExample
Output
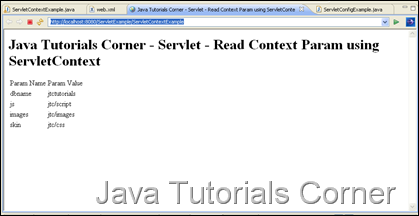
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
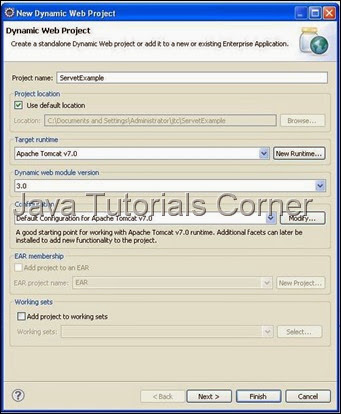
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called ServletContextExample as shown in figure.


5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
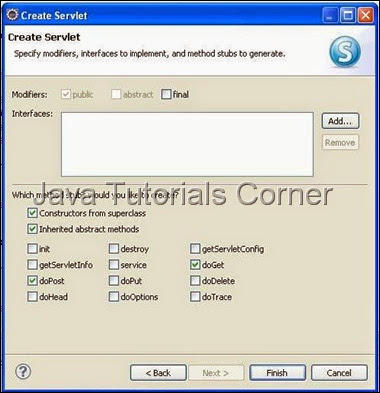
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
1.
<
servlet
>
2.
<
servlet-name
>Your Servlet Name</
servlet-name
>
3.
<
servlet-class
>Fully Qulaified Servlet Class Name</
servlet-class
>
4.
</
servlet
>
5.
<
servlet-mapping
>
6.
<
servlet-name
>Your Servlet Name</
servlet-name
>
7.
<
url-pattern
>/URL to Call Servlet</
url-pattern
>
8.
</
servlet-mapping
>
web.xml
01.
<?
xml
version
=
"1.0"
encoding
=
"UTF-8"
?>
02.
<
web-app
xmlns:xsi
=
"http://www.w3.org/2001/XMLSchema-instance"
03.
xmlns
=
"http://java.sun.com/xml/ns/javaee"
xmlns:web
=
"http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
04.
xsi:schemaLocation
=
"http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
05.
id
=
"WebApp_ID"
version
=
"3.0"
>
06.
<
display-name
>ServetExample</
display-name
>
07.
<
welcome-file-list
>
08.
<
welcome-file
>index.html</
welcome-file
>
09.
<
welcome-file
>index.htm</
welcome-file
>
10.
<
welcome-file
>index.jsp</
welcome-file
>
11.
<
welcome-file
>default.html</
welcome-file
>
12.
<
welcome-file
>default.htm</
welcome-file
>
13.
<
welcome-file
>default.jsp</
welcome-file
>
14.
</
welcome-file-list
>
15.
<
servlet
>
16.
<
servlet-name
>ServletContextExample</
servlet-name
>
17.
<
servlet-class
>com.javatutorialscorner.servlet.ServletContextExample</
servlet-class
>
18.
19.
</
servlet
>
20.
<
context-param
>
21.
<
param-name
>dbname</
param-name
>
22.
<
param-value
>jtctutorials</
param-value
>
23.
</
context-param
>
24.
<
context-param
>
25.
<
param-name
>images</
param-name
>
26.
<
param-value
>jtc/images</
param-value
>
27.
</
context-param
>
28.
<
context-param
>
29.
<
param-name
>skin</
param-name
>
30.
<
param-value
>jtc/css</
param-value
>
31.
</
context-param
>
32.
<
context-param
>
33.
<
param-name
>js</
param-name
>
34.
<
param-value
>jtc/script</
param-value
>
35.
</
context-param
>
36.
<
servlet-mapping
>
37.
<
servlet-name
>ServletContextExample</
servlet-name
>
38.
<
url-pattern
>/ServletContextExample</
url-pattern
>
39.
</
servlet-mapping
>
40.
</
web-app
>
8. Add the required code inside doGet() method.
ServletContextExample.java
01.
package
com.javatutorialscorner.servlet;
02.
03.
import
java.io.IOException;
04.
import
java.io.PrintWriter;
05.
import
java.util.Enumeration;
06.
07.
import
javax.servlet.ServletConfig;
08.
import
javax.servlet.ServletContext;
09.
import
javax.servlet.ServletException;
10.
import
javax.servlet.http.HttpServlet;
11.
import
javax.servlet.http.HttpServletRequest;
12.
import
javax.servlet.http.HttpServletResponse;
13.
14.
/**
15.
* Servlet implementation class ServletContextExample
16.
*/
17.
18.
public
class
ServletContextExample
extends
HttpServlet {
19.
private
static
final
long
serialVersionUID = 1L;
20.
21.
/**
22.
* @see HttpServlet#HttpServlet()
23.
*/
24.
public
ServletContextExample() {
25.
super
();
26.
// TODO Auto-generated constructor stub
27.
}
28.
29.
/**
30.
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
31.
* response)
32.
*/
33.
protected
void
doGet(HttpServletRequest request,
34.
HttpServletResponse response)
throws
ServletException, IOException {
35.
// TODO Auto-generated method stub
36.
37.
response.setContentType(
"text/html"
);
38.
PrintWriter writer = response.getWriter();
39.
writer.write(
"<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n"
40.
+
"<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - Read Context Param using ServletContext </title>"
41.
+
"</head><body><h1>Java Tutorials Corner - Servlet - Read Context Param using ServletContext</h1><table>"
);
42.
writer.write(
"<tr><td> Param Name </td><td> Param Value</td></tr>"
);
43.
44.
ServletContext servletContext = getServletContext();
45.
Enumeration<String> contextParams = servletContext
46.
.getInitParameterNames();
47.
48.
while
(contextParams.hasMoreElements()) {
49.
String paramName = (String) contextParams.nextElement();
50.
writer.write(
"<tr><td> "
+ paramName +
"</td><td>"
51.
+ servletContext.getInitParameter(paramName) +
"</td></tr>"
);
52.
}
53.
54.
writer.write(
"</table></body></html>"
);
55.
}
56.
57.
/**
58.
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
59.
* response)
60.
*/
61.
protected
void
doPost(HttpServletRequest request,
62.
HttpServletResponse response)
throws
ServletException, IOException {
63.
// TODO Auto-generated method stub
64.
}
65.
66.
}
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/ServletContextExample
Output
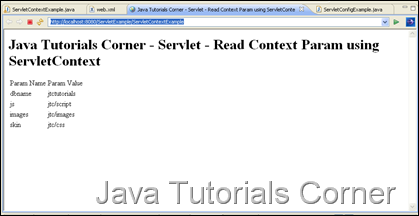
0 comments:
Post a Comment