In this tutorial we are going to see about File upload using Servlet 3 @MultipartConfig Annotation. In previous tutorial I wrote File upload using Apache File Upload API click here to see File Upload using Apache File upload API
MultipartConfig Annotation
The @MultipartConfig annotation supports the following optional attributes:
location: An absolute path to a directory on the file system. The location attribute does not support a path relative to the application context. This location is used to store files temporarily while the parts are processed or when the size of the file exceeds the specified fileSizeThreshold setting. The default location is "".
fileSizeThreshold: The file size in bytes after which the file will be temporarily stored on disk. The default size is 0 bytes.
MaxFileSize: The maximum size allowed for uploaded files, in bytes. If the size of any uploaded file is greater than this size, the web container will throw an exception (IllegalStateException). The default size is unlimited.
maxRequestSize: The maximum size allowed for a multipart/form-data request, in bytes. The web container will throw an exception if the overall size of all uploaded files exceeds this threshold. The default size is unlimited.
For, example, the @MultipartConfig annotation could be constructed as follows:
Instead of using the @MultipartConfig annotation to hard-code these attributes in your file upload servlet, you could add the following as a child element of the servlet configuration element in the web.xml file.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
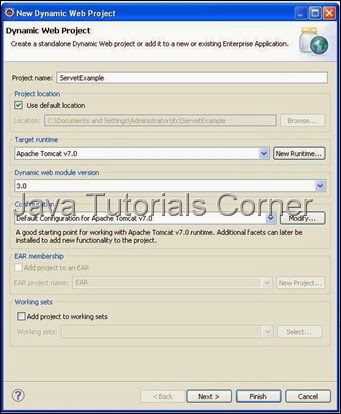
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called Servlet3FileUpload as shown in figure.
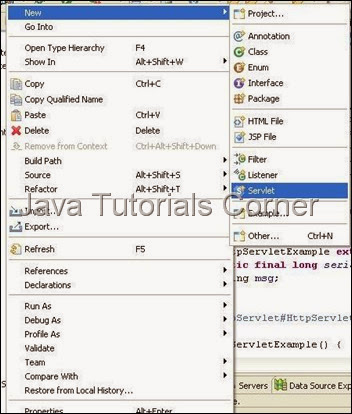
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. In Servlet 3 @WebServlet (instead of web.xml)Annotation used for URL mapping . URL mapping will be look like this.
8. Add the required code inside doPost() method.
Servlet3FileUpload.java
9. Create html page in WebContent folder
fileupload.html
In above html page in form tag method="post" to mention the form submit using post method.The default method to submit form is GET.
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the following URL .
http://localhost:8080/ServletExample/fileupload.html
Output
After File Uploaded
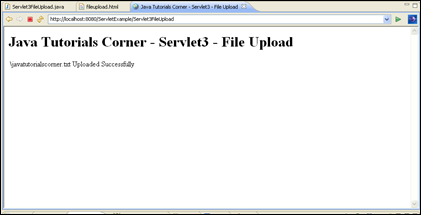
MultipartConfig Annotation
The @MultipartConfig annotation supports the following optional attributes:
location: An absolute path to a directory on the file system. The location attribute does not support a path relative to the application context. This location is used to store files temporarily while the parts are processed or when the size of the file exceeds the specified fileSizeThreshold setting. The default location is "".
fileSizeThreshold: The file size in bytes after which the file will be temporarily stored on disk. The default size is 0 bytes.
MaxFileSize: The maximum size allowed for uploaded files, in bytes. If the size of any uploaded file is greater than this size, the web container will throw an exception (IllegalStateException). The default size is unlimited.
maxRequestSize: The maximum size allowed for a multipart/form-data request, in bytes. The web container will throw an exception if the overall size of all uploaded files exceeds this threshold. The default size is unlimited.
For, example, the @MultipartConfig annotation could be constructed as follows:
1.
@MultipartConfig
(location=
"/tmp"
, fileSizeThreshold=
1024
*
1024
, maxFileSize=
1024
*
1024
*
5
, maxRequestSize=
1024
*
1024
*
5
*
5
)
Instead of using the @MultipartConfig annotation to hard-code these attributes in your file upload servlet, you could add the following as a child element of the servlet configuration element in the web.xml file.
1.
<
multipart-config
>
2.
<
location
>/tmp</
location
>
3.
<
max-file-size
>20848820</
max-file-size
>
4.
<
max-request-size
>418018841</
max-request-size
>
5.
<
file-size-threshold
>1048576</
file-size-threshold
>
6.
</
multipart-config
>
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
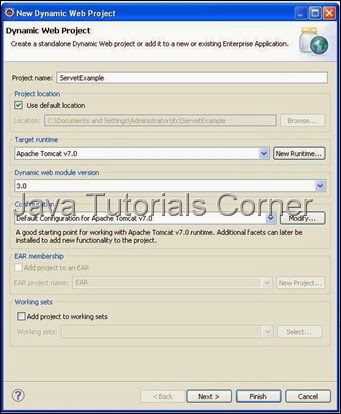
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called Servlet3FileUpload as shown in figure.
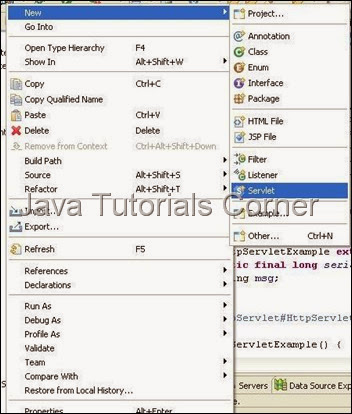

5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
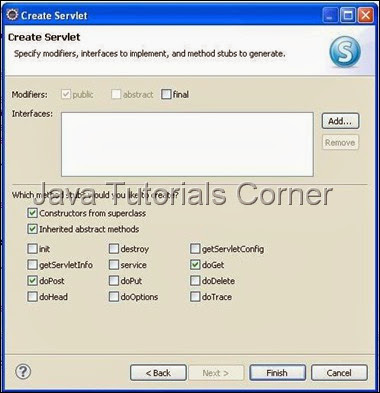
7. In Servlet 3 @WebServlet (instead of web.xml)Annotation used for URL mapping . URL mapping will be look like this.
1.
@WebServlet
(
"/Servlet3FileUpload"
)
8. Add the required code inside doPost() method.
Servlet3FileUpload.java
01.
package
com.javatutorialscorner.servlet;
02.
03.
import
java.io.File;
04.
import
java.io.IOException;
05.
import
java.io.PrintWriter;
06.
07.
import
javax.servlet.ServletException;
08.
import
javax.servlet.annotation.MultipartConfig;
09.
import
javax.servlet.annotation.WebServlet;
10.
import
javax.servlet.http.HttpServlet;
11.
import
javax.servlet.http.HttpServletRequest;
12.
import
javax.servlet.http.HttpServletResponse;
13.
import
javax.servlet.http.Part;
14.
15.
/**
16.
* Servlet implementation class Servlet3FileUpload
17.
*/
18.
@WebServlet
(
"/Servlet3FileUpload"
)
19.
@MultipartConfig
(fileSizeThreshold =
1024
*
1024
*
5
, maxFileSize =
1014
*
1024
*
25
, maxRequestSize =
1024
*
1024
*
50
)
20.
public
class
Servlet3FileUpload
extends
HttpServlet {
21.
private
static
final
long
serialVersionUID = 1L;
22.
23.
/**
24.
* @see HttpServlet#HttpServlet()
25.
*/
26.
public
Servlet3FileUpload() {
27.
super
();
28.
// TODO Auto-generated constructor stub
29.
}
30.
31.
/**
32.
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
33.
* response)
34.
*/
35.
protected
void
doGet(HttpServletRequest request,
36.
HttpServletResponse response)
throws
ServletException, IOException {
37.
// TODO Auto-generated method stub
38.
}
39.
40.
/**
41.
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
42.
* response)
43.
*/
44.
protected
void
doPost(HttpServletRequest request,
45.
HttpServletResponse response)
throws
ServletException, IOException {
46.
// TODO Auto-generated method stub
47.
String realPath = request.getServletContext().getRealPath(
""
);
48.
String destinationUpload = realPath + File.separator +
"JTC"
;
49.
String fileName =
null
;
50.
File file =
new
File(destinationUpload);
51.
if
(!file.exists())
52.
file.mkdirs();
53.
for
(Part part : request.getParts()) {
54.
fileName = getUploadedFileName(part);
55.
part.write(destinationUpload + File.separator + fileName);
56.
}
57.
response.setContentType(
"text/html"
);
58.
PrintWriter writer = response.getWriter();
59.
writer.write(
"<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n"
60.
+
"<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet3 - File Upload</title>"
61.
+
"</head><body><h1>Java Tutorials Corner - Servlet3 - File Upload</h1><table><tr><td>"
62.
+ fileName +
" Uploaded Successfully</td></tr>"
);
63.
64.
}
65.
66.
private
String getUploadedFileName(Part part) {
67.
// TODO Auto-generated method stub
68.
String header = part.getHeader(
"content-disposition"
);
69.
String[] tokens = header.split(
";"
);
70.
String fileName =
""
;
71.
for
(String headerParam : tokens) {
72.
if
(headerParam.trim().startsWith(
"filename"
)) {
73.
74.
fileName = headerParam.substring(headerParam.indexOf(
"="
) +
2
,
75.
headerParam.length() -
1
);
76.
}
77.
78.
}
79.
if
(fileName.lastIndexOf(
"\\"
) >=
0
) {
80.
81.
fileName = fileName.substring(fileName.lastIndexOf(
"\\"
));
82.
}
else
{
83.
fileName = fileName.substring(fileName.lastIndexOf(
"\\"
) +
1
);
84.
}
85.
86.
return
fileName;
87.
}
88.
89.
}
9. Create html page in WebContent folder
fileupload.html
01.
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
02.
<
html
>
03.
<
head
>
04.
<
meta
http-equiv
=
"Content-Type"
content
=
"text/html; charset=ISO-8859-1"
>
05.
<
title
>Java Tutorials Corner - Servlet3 File Upload</
title
>
06.
</
head
>
07.
<
body
>
08.
<
h1
>Java Tutorials Corner - Servlet3 File Upload</
h1
>
09.
<
form
action
=
"Servlet3FileUpload"
method
=
"post"
10.
enctype
=
"multipart/form-data"
>
11.
<
input
type
=
"file"
name
=
"file"
size
=
"50"
/>
12.
<
input
13.
type
=
"submit"
value
=
"Submit"
/>
14.
</
form
>
15.
</
body
>
16.
</
html
>
In above html page in form tag method="post" to mention the form submit using post method.The default method to submit form is GET.
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the following URL .
http://localhost:8080/ServletExample/fileupload.html
Output
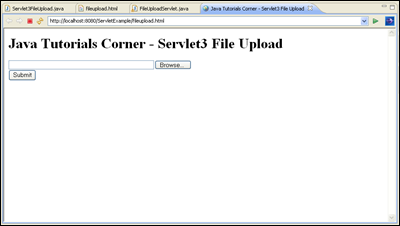
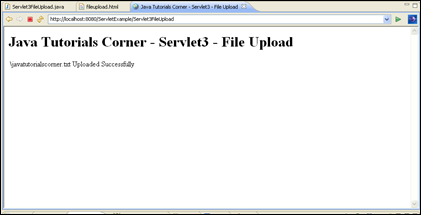
0 comments:
Post a Comment