In this tutorial we are going to see how to upload file using servlet
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called FileUploadServlet as shown in figure.
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add commons-fileupload-1.3.1.jar , commons-io-1.3.2.jar in your build path.
9. Add the required code inside doPost() method.
FileUploadServlet.java
In above program
ServletFileUpload.isMultipartContent(request); – to check multi part data present in request.
diskFileItemFactory.setSizeThreshold(2048); – maximum size that will be stored in memory.
diskFileItemFactory.setRepository(new File("C://jtc//temp")); – Temporary location to store the data that is larger than maximum size.
servletFileUpload.setSizeMax(2048); – Maximum file size to be uploaded
10. Create html page in WebContent folder
fileupload.html
In above html page in form tag method="post" to mention the form submit using post method.The default method to submit form is GET. Get cannot be used for file upload size limit restriction.
The enctype="multipart/form-data" must be specified.
10. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
11.call the following URL.
http://localhost:8080/ServletExample/fileupload.html
Output
After File uploaded
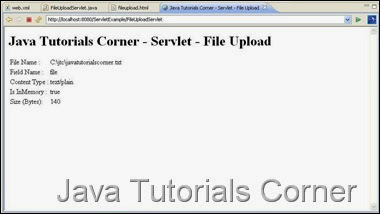
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.

3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called FileUploadServlet as shown in figure.


5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>FileUploadServlet</servlet-name> <servlet-class>com.javatutorialscorner.servlet.FileUploadServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>FileUploadServlet</servlet-name> <url-pattern>/FileUploadServlet</url-pattern> </servlet-mapping> </web-app>
8. Add commons-fileupload-1.3.1.jar , commons-io-1.3.2.jar in your build path.
9. Add the required code inside doPost() method.
FileUploadServlet.java
package com.javatutorialscorner.servlet; import java.io.File; import java.io.IOException; import java.io.PrintWriter; import java.util.Iterator; import java.util.List; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.apache.commons.fileupload.FileItem; import org.apache.commons.fileupload.FileUploadException; import org.apache.commons.fileupload.disk.DiskFileItemFactory; import org.apache.commons.fileupload.servlet.ServletFileUpload; /** * Servlet implementation class FileUploadServlet */ public class FileUploadServlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public FileUploadServlet() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub response.setContentType("text/html"); PrintWriter writer = response.getWriter(); boolean isMultipart = ServletFileUpload.isMultipartContent(request); if (!isMultipart) { writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - File Upload</title>" + "</head><body><h1>Java Tutorials Corner - Servlet - File Upload</h1><br/> File Not uploaded"); } else { writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - File Upload</title>" + "</head><body><h1>Java Tutorials Corner - Servlet - File Upload</h1><table>"); try { DiskFileItemFactory diskFileItemFactory = new DiskFileItemFactory(); diskFileItemFactory.setSizeThreshold(2048); diskFileItemFactory.setRepository(new File("C://jtc//temp")); ServletFileUpload servletFileUpload = new ServletFileUpload( diskFileItemFactory); servletFileUpload.setSizeMax(2048); List<FileItem> fileItemList = servletFileUpload .parseRequest(request); Iterator iterator = fileItemList.iterator(); while (iterator.hasNext()) { FileItem fileItem = (FileItem) iterator.next(); if (!fileItem.isFormField()) { String fieldName = fileItem.getFieldName(); String fileName = fileItem.getName(); String contentType = fileItem.getContentType(); boolean isInMemory = fileItem.isInMemory(); long size = fileItem.getSize(); writer.write("<tr><td>File Name :</td><td>" + fileName + "</td></tr>"); writer.write("<tr><td>Field Name :</td><td>" + fieldName + "</td></tr>"); writer.write("<tr><td>Content Type :</td><td>" + contentType + "</td></tr>"); writer.write("<tr><td>Is InMemory :</td><td>" + isInMemory + "</td></tr>"); writer.write("<tr><td>Size (Bytes):</td><td>" + size + "</td></tr>"); File file = null; String fileDestination = "C://jtc//"; if (fileName.lastIndexOf("\\") >= 0) { file = new File(fileDestination + fileName.substring(fileName .lastIndexOf("\\"))); } else { file = new File(fileDestination + fileName.substring(fileName .lastIndexOf("\\") + 1)); } fileItem.write(file); } } } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } writer.write("</table></body></html>"); } } }
In above program
ServletFileUpload.isMultipartContent(request); – to check multi part data present in request.
diskFileItemFactory.setSizeThreshold(2048); – maximum size that will be stored in memory.
diskFileItemFactory.setRepository(new File("C://jtc//temp")); – Temporary location to store the data that is larger than maximum size.
servletFileUpload.setSizeMax(2048); – Maximum file size to be uploaded
10. Create html page in WebContent folder
fileupload.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Java Tutorials Corner - Servlet File Upload</title> </head> <body> <h1>Java Tutorials Corner - Servlet File Upload</h1> <form action="FileUploadServlet" method="post" enctype="multipart/form-data"> <input type="file" name="file" size="50" /> <br /> <input type="submit" value="Submit" /> </form> </body> </html>
In above html page in form tag method="post" to mention the form submit using post method.The default method to submit form is GET. Get cannot be used for file upload size limit restriction.
The enctype="multipart/form-data" must be specified.
10. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
11.call the following URL.
http://localhost:8080/ServletExample/fileupload.html
Output

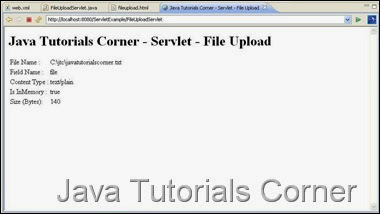
0 comments:
Post a Comment