In this tutorial we are going to see how to read header information from servlet request with example program. Click here to read more about HTTP/1.1 Header param with Definitions
Methods to read header value
public long getDateHeader(java.lang.String name)
Returns the value of the specified request header as a long value that represents a Date object. Use this method with headers that contain dates, such as If-Modified-Since.
The date is returned as the number of milliseconds since January 1, 1970 GMT. The header name is case insensitive.
If the request did not have a header of the specified name, this method returns -1. If the header can't be converted to a date, the method throws an IllegalArgumentException.
public java.lang.String getHeader(java.lang.String name)
Returns the value of the specified request header as a String. If the request did not include a header of the specified name, this method returns null. If there are multiple headers with the same name, this method returns the first head in the request. The header name is case insensitive. You can use this method with any request header.
public java.util.Enumeration getHeaders(java.lang.String name)
Returns all the values of the specified request header as an Enumeration of String objects.
Some headers, such as Accept-Language can be sent by clients as several headers each with a different value rather than sending the header as a comma separated list.
If the request did not include any headers of the specified name, this method returns an empty Enumeration. The header name is case insensitive. You can use this method with any request header.
public java.util.Enumeration getHeaderNames()
Returns an enumeration of all the header names this request contains. If the request has no headers, this method returns an empty enumeration.
Some servlet containers do not allow servlets to access headers using this method, in which case this method returns null
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
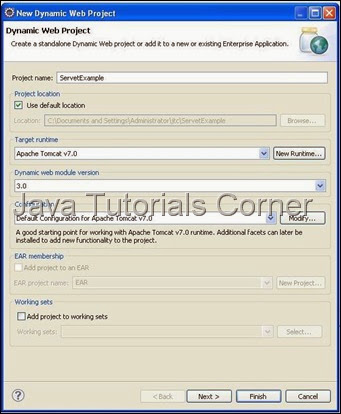
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called HttpRequestHeader as shown in figure.
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add the required code inside doGet() method.
HttpRequestHeader.java
In above servlet request.getHeaderNames() is used to read header information from request, This method returns Enumeration of header values.
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/HttpRequestHeader
Output
Header Name Header Value
accept */*
accept-language en-us
accept-encoding gzip, deflate
user-agent Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1; BTRS100277;InfoPath.2; .NET CLR 2.0.50727; .NET CLR 3.0.04506.648; .NET CLR 3.5.21022)
host ocalhost:8080
connection Keep-Alive
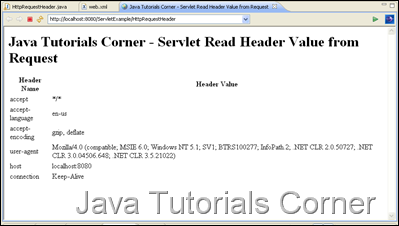
Headers
|
Descriptions
|
Accept | This headed used to specify MIME types that client/browser can handle. example image/png , image/jpeg |
Accept-Charset | This headed used to specify the character set that the browser used to display information. Example ISO-8859-1 |
Accept-Encoding | This headed used to specify the type of encoding that the browser know how to handle. example gzip or compress |
Accept-Language | This headed used to specify the client prepared language.example en, en-us, ru, etc |
Authorization | This headed used by the client to identify themselves when accessing the password protected web pages. |
Connection | This header indicates whether the client can handle persistent HTTP connections. Persistent connections permit the client or other browser to retrieve multiple files with a single request. A value of Keep-Alive means that persistent connections should be used |
Content-Length | This header applicable only for POST request. This is used to specify the size of POST data in bytes. |
Cookie | This header returns cookies to servlet that previously sent to browser. |
Host | This header specifies the host and port as given in original URL |
User-Agent | This header identifies the client or browser which making the request |
If-Modified-Since | This header indicates that the client wants the page only if it has been changed after the specified date. The server sends a code, 304 which means Not Modified header if no newer result is available |
If-Unmodified-Since | This header is the reverse of If-Modified-Since; it specifies that the operation should succeed only if the document is older than the specified date. |
Refer | This header indicates the URL of the referring Web page. |
public long getDateHeader(java.lang.String name)
Returns the value of the specified request header as a long value that represents a Date object. Use this method with headers that contain dates, such as If-Modified-Since.
The date is returned as the number of milliseconds since January 1, 1970 GMT. The header name is case insensitive.
If the request did not have a header of the specified name, this method returns -1. If the header can't be converted to a date, the method throws an IllegalArgumentException.
public java.lang.String getHeader(java.lang.String name)
Returns the value of the specified request header as a String. If the request did not include a header of the specified name, this method returns null. If there are multiple headers with the same name, this method returns the first head in the request. The header name is case insensitive. You can use this method with any request header.
public java.util.Enumeration getHeaders(java.lang.String name)
Returns all the values of the specified request header as an Enumeration of String objects.
Some headers, such as Accept-Language can be sent by clients as several headers each with a different value rather than sending the header as a comma separated list.
If the request did not include any headers of the specified name, this method returns an empty Enumeration. The header name is case insensitive. You can use this method with any request header.
public java.util.Enumeration getHeaderNames()
Returns an enumeration of all the header names this request contains. If the request has no headers, this method returns an empty enumeration.
Some servlet containers do not allow servlets to access headers using this method, in which case this method returns null
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
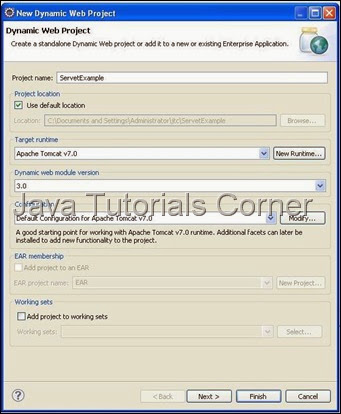
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called HttpRequestHeader as shown in figure.


5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>HttpRequestHeader</servlet-name> <servlet-class>com.javatutorialscorner.servlet.HttpRequestHeader</servlet-class> </servlet> <servlet-mapping> <servlet-name>HttpRequestHeader</servlet-name> <url-pattern>/HttpRequestHeader</url-pattern> </servlet-mapping> </web-app>
8. Add the required code inside doGet() method.
HttpRequestHeader.java
package com.javatutorialscorner.servlet; import java.io.IOException; import java.io.PrintWriter; import java.util.Enumeration; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class HttpRequestHeader */ public class HttpRequestHeader extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public HttpRequestHeader() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub response.setContentType("text/html"); PrintWriter writer = response.getWriter(); writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet Read Header Value from Request</title>" + "</head><body><h1>Java Tutorials Corner - Servlet Read Header Value from Request</h1><table><tr>" + "<th>Header Name </td><th> Header Value</th></tr>"); Enumeration headers = request.getHeaderNames(); while (headers.hasMoreElements()) { String headerParam = (String) headers.nextElement(); writer.write("<tr><td>" + headerParam + "</td><td>" + request.getHeader(headerParam) + "</td></tr>"); } writer.write("</table></body></html>"); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
In above servlet request.getHeaderNames() is used to read header information from request, This method returns Enumeration of header values.
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/HttpRequestHeader
Output
Header Name Header Value
accept */*
accept-language en-us
accept-encoding gzip, deflate
user-agent Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1; BTRS100277;InfoPath.2; .NET CLR 2.0.50727; .NET CLR 3.0.04506.648; .NET CLR 3.5.21022)
host ocalhost:8080
connection Keep-Alive
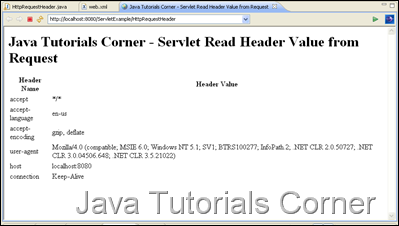
0 comments:
Post a Comment