In this tutorial we are going to see about ServletContext Interface with example program.
public interface ServletContext
Defines a set of methods that a servlet uses to communicate with its servlet container, for example, to get the MIME type of a file, dispatch requests, write to a log file. Simple ServletContext object can be used to provide inter-application communication like pass configuration information from web.xml, set, get, remove attributes in application scope etc.
There is one context per "web application" per Java Virtual Machine.
The ServletContext object is contained within the ServletConfig object, which the Web server provides the servlet when the servlet is initialized.
The getServletContext(); method of ServletConfig interface returns ServletContext object.
Fields in ServletContext Interface
static final String TEMPDIR
The name of the ServletContext attribute which stores the private temporary directory (of type java.io.File) provided by the servlet container for the ServletContext
static final String ORDERED_LIBS
The name of the ServletContext attribute whose value (of type java.util.List<java.lang.String>) contains the list of names of JAR files in WEB-INF/lib ordered by their web fragment names (with possible exclusions if <absolute-ordering> without any <others/> is being used), or null if no absolute or relative ordering has been specified
Methods in ServletContext Interface
Few commonly used methods alone given here. see more about Servlet Context methods
Now see ServletContext with example program.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called ServletContextExample as shown in figure.
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
<context-param>..</context-param>…
<context-param> is sub element of web-app, which is used to define initialization parameter in the application scope. The param-name , param-value are the sub element of context-param, these element used set param name and values.
8. Add the required code inside doGet() method.
ServletContextExample.java
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/ServletContextExample
Output
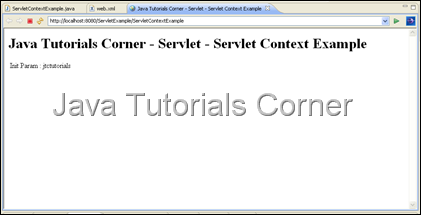
public interface ServletContext
Defines a set of methods that a servlet uses to communicate with its servlet container, for example, to get the MIME type of a file, dispatch requests, write to a log file. Simple ServletContext object can be used to provide inter-application communication like pass configuration information from web.xml, set, get, remove attributes in application scope etc.
There is one context per "web application" per Java Virtual Machine.
The ServletContext object is contained within the ServletConfig object, which the Web server provides the servlet when the servlet is initialized.
The getServletContext(); method of ServletConfig interface returns ServletContext object.
Fields in ServletContext Interface
static final String TEMPDIR
The name of the ServletContext attribute which stores the private temporary directory (of type java.io.File) provided by the servlet container for the ServletContext
static final String ORDERED_LIBS
The name of the ServletContext attribute whose value (of type java.util.List<java.lang.String>) contains the list of names of JAR files in WEB-INF/lib ordered by their web fragment names (with possible exclusions if <absolute-ordering> without any <others/> is being used), or null if no absolute or relative ordering has been specified
Methods in ServletContext Interface
Few commonly used methods alone given here. see more about Servlet Context methods
S.NO |
Method with Description
|
1 | ServletContext getContext(String uripath) Returns a ServletContext object that corresponds to a specified URL on the server. |
2 | String getContextPath() Returns the context path of the web application. |
3 | RequestDispatcher getRequestDispatcher(String path) Returns a RequestDispatcher object that acts as a wrapper for the resource located at the given path. A RequestDispatcher object can be used to forward a request to the resource or to include the resource in a response. The resource can be dynamic or static. |
4 | String getInitParameter(String name) Returns a String containing the value of the named context-wide initialization parameter, or null if the parameter does not exist. |
5 | java.util.Enumeration<String> getInitParameterNames() Returns the names of the context's initialization parameters as an Enumeration of String objects, or an empty Enumeration if the context has no initialization parameters. |
6 | Object getAttribute(String name) Returns the servlet container attribute with the given name, or null if there is no attribute by that name |
7 | java.util.Enumeration<String> getAttributeNames() Returns an Enumeration containing the attribute names available within this ServletContext. |
8 | void setAttribute(java.lang.String name,java.lang.Object object) Binds an object to a given attribute name in this ServletContext. If the name specified is already used for an attribute, this method will replace the attribute with the new to the new attribute. |
9 | void removeAttribute(String name) Removes the attribute with the given name from this ServletContext. After removal, subsequent calls to getAttribute(String) to retrieve the attribute's value will return null. |
Now see ServletContext with example program.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.

3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called ServletContextExample as shown in figure.
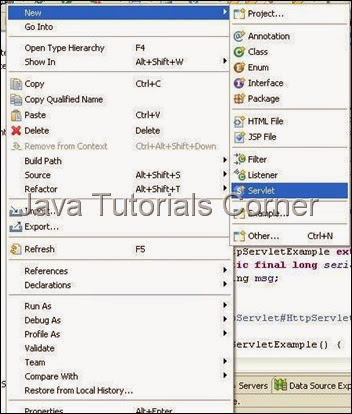

5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>ServletContextExample</servlet-name> <servlet-class>com.javatutorialscorner.servlet.ServletContextExample</servlet-class> </servlet> <context-param> <param-name>dbname</param-name> <param-value>jtctutorials</param-value> </context-param> <servlet-mapping> <servlet-name>ServletContextExample</servlet-name> <url-pattern>/ServletContextExample</url-pattern> </servlet-mapping> </web-app>
<context-param>..</context-param>…
<context-param> is sub element of web-app, which is used to define initialization parameter in the application scope. The param-name , param-value are the sub element of context-param, these element used set param name and values.
8. Add the required code inside doGet() method.
ServletContextExample.java
package com.javatutorialscorner.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletContext; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class ServletContextExample */ public class ServletContextExample extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public ServletContextExample() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub ServletContext servletContext = getServletContext(); String contextParam = servletContext.getInitParameter("dbname"); response.setContentType("text/html"); PrintWriter writer = response.getWriter(); writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - Servlet Context Example</title>" + "</head><body><h1>Java Tutorials Corner - Servlet - Servlet Context Example</h1><table><tr>" + "<td> Init Param : </td><td>" + contextParam + "</td></tr></table></body></html>"); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/ServletContextExample
Output
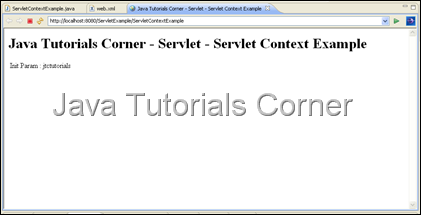
0 comments:
Post a Comment