In this tutorial we are going to see about Exception Handling in Servlet with example program.
Servlet Exception Handling
In general doGet(), doPost() methods of servlet throws ServletException, IOException. When a servlet throws exception, the web container search the configuration in web.xml for exception handling.
The <error-page> tag in web.xl used to handle exception. The error type matched using <exception-type> or <error-code>403</error-code> , these elements are sub element of <error-page>.
Example error handling using error-code
Example error handling for single exception-type
Example error handling for generic exception-type
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project.
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called ServletExceptionHandling as shown in figure.
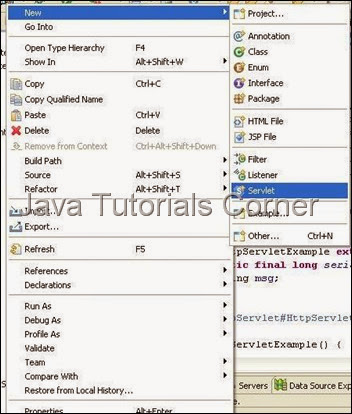
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add the required code inside goGet() method.
ServletExceptionHandling.java
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the following URL which is not mapped in web.xml, so it will throw 404 exception.
http://localhost:8080/ServletExample/Helloworld
Output
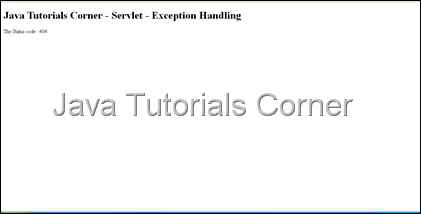
Servlet Exception Handling
In general doGet(), doPost() methods of servlet throws ServletException, IOException. When a servlet throws exception, the web container search the configuration in web.xml for exception handling.
The <error-page> tag in web.xl used to handle exception. The error type matched using <exception-type> or <error-code>403</error-code> , these elements are sub element of <error-page>.
Example error handling using error-code
<error-page> <error-code>403</error-code> <location>/ServletExceptionHandling</location> </error-page>
Example error handling for single exception-type
<error-page> <exception-type>java.io.IOException</exception-type> <location>/ServletExceptionHandling</location> </error-page>
Example error handling for generic exception-type
<error-page> <exception-type>java.lang.Throwable</exception-type> <location>/ServletExceptionHandling</location> </error-page>
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project.
2. Create the Project called ServletExample as given below.

3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called ServletExceptionHandling as shown in figure.
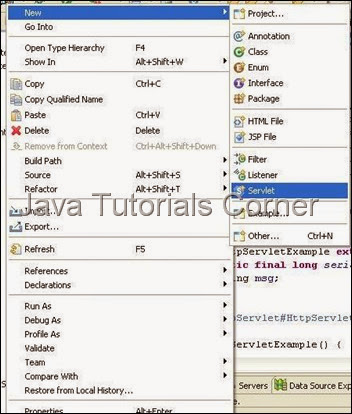

5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>ServletExceptionHandling</servlet-name> <servlet-class>com.javatutorialscorner.servlet.ServletExceptionHandling</servlet-class> </servlet> <servlet-mapping> <servlet-name>ServletExceptionHandling</servlet-name> <url-pattern>/ServletExceptionHandling</url-pattern> </servlet-mapping> <!-- Error code and error page --> <error-page> <exception-type>java.io.IOException</exception-type> <location>/ServletExceptionHandling</location> </error-page> <error-page> <exception-type>javax.servlet.ServletException</exception-type> <location>/ServletExceptionHandling</location> </error-page> <!-- error-code and error page --> <error-page> <error-code>403</error-code> <location>/ServletExceptionHandling</location> </error-page> <error-page> <error-code>404</error-code> <location>/ServletExceptionHandling</location> </error-page> </web-app>
8. Add the required code inside goGet() method.
ServletExceptionHandling.java
package com.javatutorialscorner.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class ServletExceptionHandling */ public class ServletExceptionHandling extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public ServletExceptionHandling() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub Throwable exThrowable = (Throwable) request .getAttribute("javax.servlet.error.exception"); Integer errorcode = (Integer) request .getAttribute("javax.servlet.error.status_code"); String servletName = (String) request .getAttribute("javax.servlet.error.servlet_name"); String requestUri = (String) request .getAttribute("javax.servlet.error.request_uri"); if (servletName == null) { servletName = "Unknown"; } if (requestUri == null) { requestUri = "Unknown"; } response.setContentType("text/html"); PrintWriter writer = response.getWriter(); writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - Exception Handling</title>" + "</head><body><h1>Java Tutorials Corner - Servlet - Exception Handling</h1>"); if (exThrowable == null && errorcode == null) { writer.println("<h2>Error details missing</h2><br/> please go back to home <a href=\"" + response.encodeURL("http://localhost:8080/") + "\">Home Page</a>."); } else if (errorcode != null) { writer.println("The Status code : " + errorcode); } else { writer.println("<h2>Error Info</h2>"); writer.println("Servlet Name : " + servletName + "</br></br>"); writer.println("Exception Type : " + exThrowable.getClass().getName() + "</br></br>"); writer.println("The request URI: " + requestUri + "<br><br>"); writer.println("The exception message: " + exThrowable.getMessage()); } writer.write("</body></html>"); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the following URL which is not mapped in web.xml, so it will throw 404 exception.
http://localhost:8080/ServletExample/Helloworld
Output
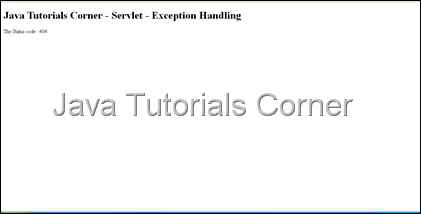
0 comments:
Post a Comment