In this tutorial we are going to see about Servlet Request Dispatcher include with example program.
Includes the content of a resource (servlet, JSP page, HTML file) in the response. In essence, this method enables programmatic server-side includes.
The ServletResponse object has its path elements and parameters remain unchanged from the caller's. The included servlet cannot change the response status code or set headers; any attempt to make a change is ignored.
The request and response parameters must be either the same objects as were passed to the calling servlet's service method or be subclasses of the ServletRequestWrapper or ServletResponseWrapper classes that wrap them.
This method sets the dispatcher type of the given request to DispatcherType.INCLUDE.
request - a ServletRequest object that contains the client's request
response - a ServletResponse object that contains the servlet's response
The getRequestDispatcher(String) method of request object returns RequestDispatcher Interface.
Now see the example program
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create following Servlet as shown in figure.
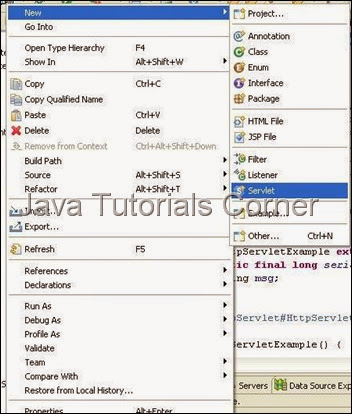
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add the required code inside doGet() method.
RequestDispatcherInclude.java
DestinationServlet.java
9. Create html page in WebContent folder
index.html
In above html page in form tag method="get"to mention the form submit using get method.The default method to submit form is GET.
10. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
11.call the following URL .
http://localhost:8080/ServletExample/index.html
Output
Click The Link page
See the response of servlet Included in page.
Click The Link Servlet
See the response of first servlet Included in Response of second Servlet.
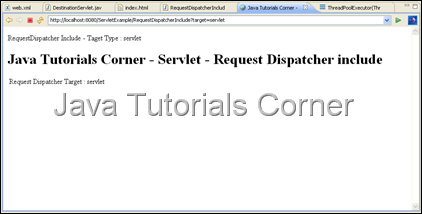
1.
void
include(ServletRequest request,ServletResponse response)
throws
ServletException,java.io.IOException
Includes the content of a resource (servlet, JSP page, HTML file) in the response. In essence, this method enables programmatic server-side includes.
The ServletResponse object has its path elements and parameters remain unchanged from the caller's. The included servlet cannot change the response status code or set headers; any attempt to make a change is ignored.
The request and response parameters must be either the same objects as were passed to the calling servlet's service method or be subclasses of the ServletRequestWrapper or ServletResponseWrapper classes that wrap them.
This method sets the dispatcher type of the given request to DispatcherType.INCLUDE.
1.
RequestDispatcher requestDispatcher = request.getRequestDispatcher(
"/index.html"
);
2.
requestDispatcher.include(request, response);
request - a ServletRequest object that contains the client's request
response - a ServletResponse object that contains the servlet's response
1.
RequestDispatcher getRequestDispatcher(String);
The getRequestDispatcher(String) method of request object returns RequestDispatcher Interface.
Now see the example program
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.

3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create following Servlet as shown in figure.
- RequestDispatcherInclude
- DestinationServlet
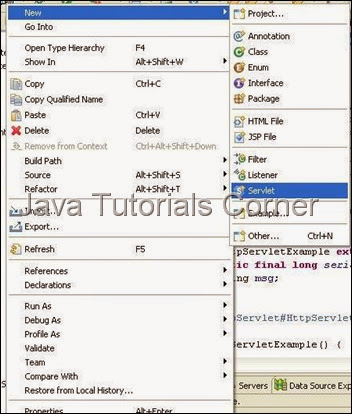
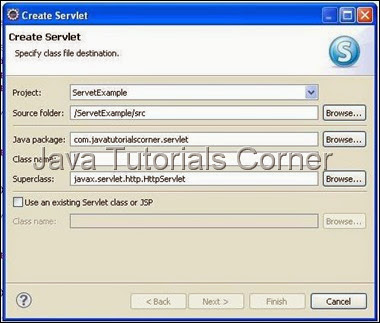
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
1.
<
servlet
>
2.
<
servlet-name
>Your Servlet Name</
servlet-name
>
3.
<
servlet-class
>Fully Qulaified Servlet Class Name</
servlet-class
>
4.
</
servlet
>
5.
<
servlet-mapping
>
6.
<
servlet-name
>Your Servlet Name</
servlet-name
>
7.
<
url-pattern
>/URL to Call Servlet</
url-pattern
>
8.
</
servlet-mapping
>
web.xml
01.
<?
xml
version
=
"1.0"
encoding
=
"UTF-8"
?>
02.
<
web-app
xmlns:xsi
=
"http://www.w3.org/2001/XMLSchema-instance"
03.
xmlns
=
"http://java.sun.com/xml/ns/javaee"
xmlns:web
=
"http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
04.
xsi:schemaLocation
=
"http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
05.
id
=
"WebApp_ID"
version
=
"3.0"
>
06.
<
display-name
>ServetExample</
display-name
>
07.
<
welcome-file-list
>
08.
<
welcome-file
>index.html</
welcome-file
>
09.
<
welcome-file
>index.htm</
welcome-file
>
10.
<
welcome-file
>index.jsp</
welcome-file
>
11.
<
welcome-file
>default.html</
welcome-file
>
12.
<
welcome-file
>default.htm</
welcome-file
>
13.
<
welcome-file
>default.jsp</
welcome-file
>
14.
</
welcome-file-list
>
15.
<
servlet
>
16.
<
servlet-name
>RequestDispatcherInclude</
servlet-name
>
17.
<
servlet-class
>com.javatutorialscorner.servlet.RequestDispatcherInclude</
servlet-class
>
18.
</
servlet
>
19.
<
servlet-mapping
>
20.
<
servlet-name
>RequestDispatcherInclude</
servlet-name
>
21.
<
url-pattern
>/RequestDispatcherInclude</
url-pattern
>
22.
</
servlet-mapping
>
23.
<
servlet
>
24.
<
servlet-name
>DestinationServlet</
servlet-name
>
25.
<
servlet-class
>com.javatutorialscorner.servlet.DestinationServlet</
servlet-class
>
26.
</
servlet
>
27.
<
servlet-mapping
>
28.
<
servlet-name
>DestinationServlet</
servlet-name
>
29.
<
url-pattern
>/DestinationServlet</
url-pattern
>
30.
</
servlet-mapping
>
31.
</
web-app
>
8. Add the required code inside doGet() method.
RequestDispatcherInclude.java
01.
package
com.javatutorialscorner.servlet;
02.
03.
import
java.io.IOException;
04.
import
java.io.PrintWriter;
05.
06.
import
javax.servlet.RequestDispatcher;
07.
import
javax.servlet.ServletException;
08.
import
javax.servlet.http.HttpServlet;
09.
import
javax.servlet.http.HttpServletRequest;
10.
import
javax.servlet.http.HttpServletResponse;
11.
12.
/**
13.
* Servlet implementation class RequestDispatcherInclude
14.
*/
15.
16.
public
class
RequestDispatcherInclude
extends
HttpServlet {
17.
private
static
final
long
serialVersionUID = 1L;
18.
19.
/**
20.
* @see HttpServlet#HttpServlet()
21.
*/
22.
public
RequestDispatcherInclude() {
23.
super
();
24.
// TODO Auto-generated constructor stub
25.
}
26.
27.
/**
28.
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
29.
* response)
30.
*/
31.
protected
void
doGet(HttpServletRequest request,
32.
HttpServletResponse response)
throws
ServletException, IOException {
33.
// TODO Auto-generated method stub
34.
String target = request.getParameter(
"target"
);
35.
RequestDispatcher requestDispatcher =
null
;
36.
response.setContentType(
"text/html"
);
37.
PrintWriter writer = response.getWriter();
38.
if
(target.equals(
"page"
)) {
39.
writer.print(
"Taget Type : "
+ target);
40.
requestDispatcher = request.getRequestDispatcher(
"/index.html"
);
41.
}
else
{
42.
writer.print(
"RequestDispatcher Include - Taget Type : "
+ target);
43.
requestDispatcher = request
44.
.getRequestDispatcher(
"DestinationServlet"
);
45.
}
46.
47.
requestDispatcher.include(request, response);
48.
49.
}
50.
51.
/**
52.
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
53.
* response)
54.
*/
55.
protected
void
doPost(HttpServletRequest request,
56.
HttpServletResponse response)
throws
ServletException, IOException {
57.
// TODO Auto-generated method stub
58.
}
59.
60.
}
DestinationServlet.java
01.
package
com.javatutorialscorner.servlet;
02.
03.
import
java.io.IOException;
04.
import
java.io.PrintWriter;
05.
06.
import
javax.servlet.ServletException;
07.
import
javax.servlet.http.HttpServlet;
08.
import
javax.servlet.http.HttpServletRequest;
09.
import
javax.servlet.http.HttpServletResponse;
10.
11.
/**
12.
* Servlet implementation class DestinationServlet
13.
*/
14.
15.
public
class
DestinationServlet
extends
HttpServlet {
16.
private
static
final
long
serialVersionUID = 1L;
17.
18.
/**
19.
* @see HttpServlet#HttpServlet()
20.
*/
21.
public
DestinationServlet() {
22.
super
();
23.
// TODO Auto-generated constructor stub
24.
}
25.
26.
/**
27.
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
28.
* response)
29.
*/
30.
protected
void
doGet(HttpServletRequest request,
31.
HttpServletResponse response)
throws
ServletException, IOException {
32.
// TODO Auto-generated method stub
33.
String target = request.getParameter(
"target"
);
34.
response.setContentType(
"text/html"
);
35.
PrintWriter writer = response.getWriter();
36.
writer.write(
"<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n"
37.
+
"<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - Request Dispatcher include</title>"
38.
+
"</head><body><h1>Java Tutorials Corner - Servlet - Request Dispatcher include</h1><table><tr>"
39.
+
"<td> Request Dispatcher Target : </td><td>"
40.
+ target
41.
+
"</td></tr></table></body></html>"
);
42.
}
43.
44.
/**
45.
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
46.
* response)
47.
*/
48.
protected
void
doPost(HttpServletRequest request,
49.
HttpServletResponse response)
throws
ServletException, IOException {
50.
// TODO Auto-generated method stub
51.
}
52.
53.
}
9. Create html page in WebContent folder
index.html
01.
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
02.
<
html
>
03.
<
head
>
04.
<
meta
http-equiv
=
"Content-Type"
content
=
"text/html; charset=ISO-8859-1"
>
05.
<
title
>Java Tutorials Corner - Servlet - Request Dispatcher
06.
Include</
title
>
07.
</
head
>
08.
<
body
>
09.
<
h1
>Java Tutorials Corner - Servlet - Request Dispatcher Include</
h1
>
10.
11.
12.
<
a
13.
href
=
"http://localhost:8080/ServletExample/RequestDispatcherInclude?target=page"
>Page</
a
>
14.
15.
16.
<
a
17.
href
=
"http://localhost:8080/ServletExample/RequestDispatcherInclude?target=servlet"
>Servlet</
a
>
18.
19.
</
body
>
20.
</
html
>
In above html page in form tag method="get"to mention the form submit using get method.The default method to submit form is GET.
10. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
11.call the following URL .
http://localhost:8080/ServletExample/index.html
Output
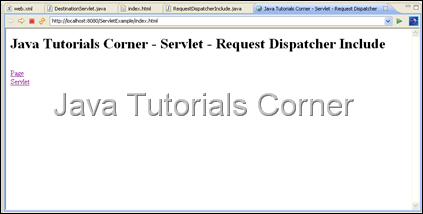
Click The Link page
See the response of servlet Included in page.
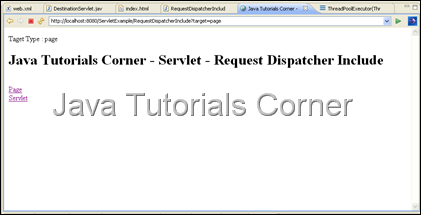
Click The Link Servlet
See the response of first servlet Included in Response of second Servlet.
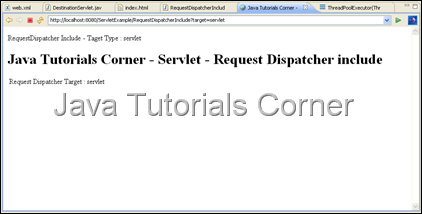
0 comments:
Post a Comment