In this tutorial we are going to see about how to count the number page view for single web page using Servlet.
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called PageViewCounterServlet as shown in figure.
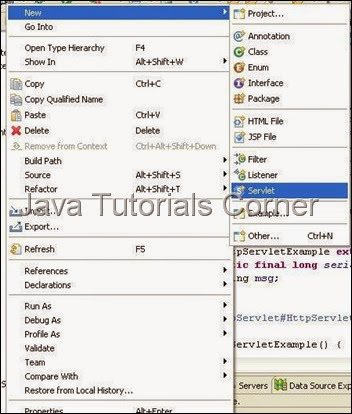
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add the required code inside doGet() method.
PageViewCounterServlet.java
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/PageViewCounterServlet
Output
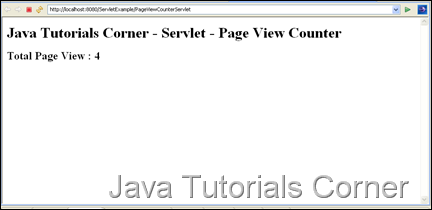
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
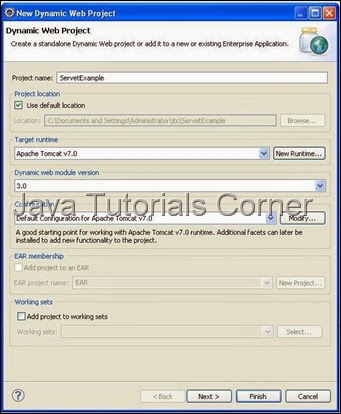
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create Servlet called PageViewCounterServlet as shown in figure.
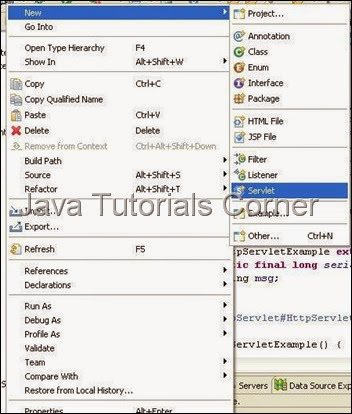

5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.

7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> </welcome-file-list> <servlet> <servlet-name>PageViewCounterServlet</servlet-name> <servlet-class>com.javatutorialscorner.servlet.PageViewCounterServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>PageViewCounterServlet</servlet-name> <url-pattern>/PageViewCounterServlet</url-pattern> </servlet-mapping> </web-app>
8. Add the required code inside doGet() method.
PageViewCounterServlet.java
package com.javatutorialscorner.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletContext; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class ServletContextGetAttribute */ public class ServletContextGetAttribute extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public ServletContextGetAttribute() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub ServletContext servletContext = getServletContext(); String contextAttribute = (String) servletContext .getAttribute("Tutorial"); response.setContentType("text/html"); PrintWriter writer = response.getWriter(); writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - Servlet Context Attribute</title>" + "</head><body><h1>Java Tutorials Corner - Servlet - Servlet Context Attribute</h1><table><tr>" + "<td> Context Attribute : </td><td>" + contextAttribute + "</td></tr></table></body></html>"); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/PageViewCounterServlet
Output
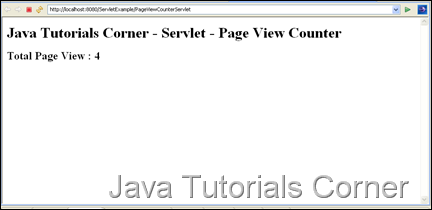
0 comments:
Post a Comment