In this tutorials we are going to see about Servlet Request Dispatcher forward with example program.
Forwards a request from a servlet to another resource (servlet, JSP file, or HTML file) on the server. This method allows one servlet to do preliminary processing of a request and another resource to generate the response.
For a RequestDispatcher obtained via getRequestDispatcher(), the ServletRequest object has its path elements and parameters adjusted to match the path of the target resource.
forward should be called before the response has been committed to the client (before response body output has been flushed). If the response already has been committed, this method throws an IllegalStateException. Uncommitted output in the response buffer is automatically cleared before the forward.
The request and response parameters must be either the same objects as were passed to the calling servlet's service method or be subclasses of the ServletRequestWrapper or ServletResponseWrapper classes that wrap them.
request - a ServletRequest object that represents the request the client makes of the servlet
response - a ServletResponse object that represents the response the servlet returns to the client
The getRequestDispatcher(String) method of request object returns RequestDispatcher Interface.
Now see the example program
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create the following Servlets as shown in figure.
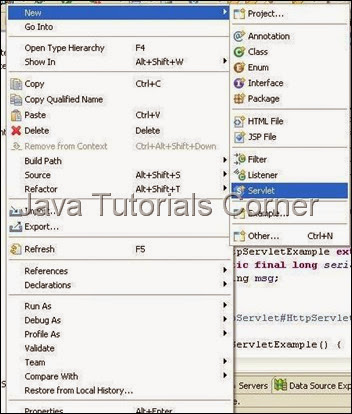
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
web.xml
8. Add the required code inside doGet() method.
RequestDispatcherForward.java
DestinationServlet.java
9. Create html page in WebContent folder
index.html
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/RequestDispatcherForward?target=servlet
Output
target=servlet
target=page
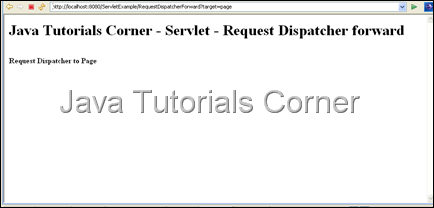
void forward(ServletRequest request,ServletResponse response)throws ServletException,java.io.IOException
Forwards a request from a servlet to another resource (servlet, JSP file, or HTML file) on the server. This method allows one servlet to do preliminary processing of a request and another resource to generate the response.
For a RequestDispatcher obtained via getRequestDispatcher(), the ServletRequest object has its path elements and parameters adjusted to match the path of the target resource.
RequestDispatcher requestDispatcher = request.getRequestDispatcher("/index.html"); equestDispatcher.forward(request, response);
forward should be called before the response has been committed to the client (before response body output has been flushed). If the response already has been committed, this method throws an IllegalStateException. Uncommitted output in the response buffer is automatically cleared before the forward.
The request and response parameters must be either the same objects as were passed to the calling servlet's service method or be subclasses of the ServletRequestWrapper or ServletResponseWrapper classes that wrap them.
request - a ServletRequest object that represents the request the client makes of the servlet
response - a ServletResponse object that represents the response the servlet returns to the client
RequestDispatcher getRequestDispatcher(String);
The getRequestDispatcher(String) method of request object returns RequestDispatcher Interface.
Now see the example program
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called ServletExample as given below.
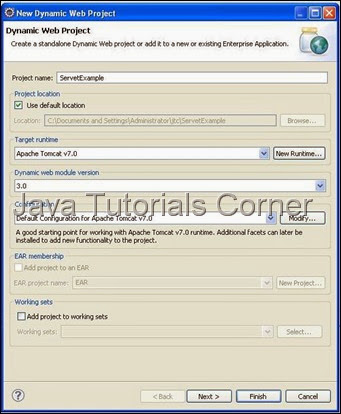
3. Create package called com.javatutorialscorner.servlet under ServletExample.
4. Create the following Servlets as shown in figure.
- RequestDispatcherForward
- DestinationServlet
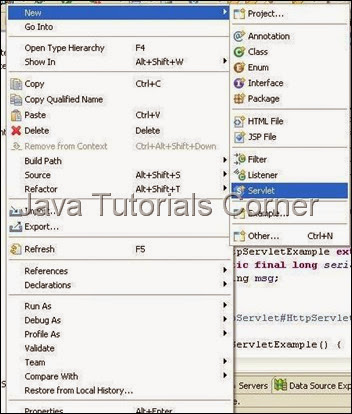
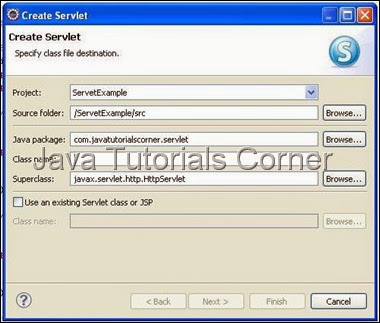
5. Click Next it will show URL mapping.You can edit Servlet URL if you need.
6. Click Next it will show methods available in HttpServlet. Select appropriate method you need.
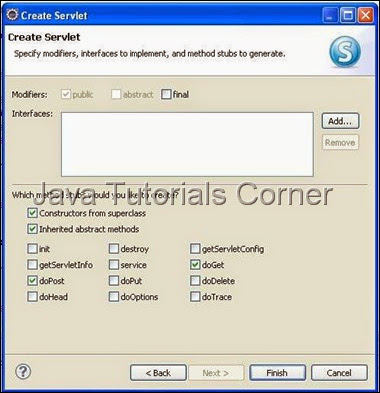
7. By default your servlet will be mapped in web.xml, if your servlet not mapped in your web.xml use the following configuration to map the servlet in your web.xml
Servlet Mapping
<servlet> <servlet-name>Your Servlet Name</servlet-name> <servlet-class>Fully Qulaified Servlet Class Name</servlet-class> </servlet> <servlet-mapping> <servlet-name>Your Servlet Name</servlet-name> <url-pattern>/URL to Call Servlet</url-pattern> </servlet-mapping>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>ServetExample</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>RequestDispatcherForward</servlet-name> <servlet-class>com.javatutorialscorner.servlet.RequestDispatcherForward</servlet-class> </servlet> <servlet-mapping> <servlet-name>RequestDispatcherForward</servlet-name> <url-pattern>/RequestDispatcherForward</url-pattern> </servlet-mapping> <servlet> <servlet-name>DestinationServlet</servlet-name> <servlet-class>com.javatutorialscorner.servlet.DestinationServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>DestinationServlet</servlet-name> <url-pattern>/DestinationServlet</url-pattern> </servlet-mapping> </web-app>
8. Add the required code inside doGet() method.
RequestDispatcherForward.java
package com.javatutorialscorner.servlet; import java.io.IOException; import javax.servlet.RequestDispatcher; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class RequestDispatcherForward */ public class RequestDispatcherForward extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public RequestDispatcherForward() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub String target = request.getParameter("target"); RequestDispatcher requestDispatcher = null; if (target.equals("page")) { requestDispatcher = request.getRequestDispatcher("/index.html"); } else { requestDispatcher = request .getRequestDispatcher("DestinationServlet"); } requestDispatcher.forward(request, response); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
DestinationServlet.java
package com.javatutorialscorner.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * Servlet implementation class DestinationServlet */ public class DestinationServlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public DestinationServlet() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse * response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub String target = request.getParameter("target"); response.setContentType("text/html"); PrintWriter writer = response.getWriter(); writer.write("<!DOCTYPE html PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\" \"http://www.w3.org/TR/html4/loose.dtd\">\n" + "<html><head><meta http-equiv=\"Content-Type\" content=\"text/html; charset=ISO-8859-1\"><title>Java Tutorials Corner - Servlet - Request Dispatcher forward</title>" + "</head><body><h1>Java Tutorials Corner - Servlet - Request Dispatcher forward</h1><table><tr>" + "<td> Request Dispatcher Target : </td><td>" + target + "</td></tr></table></body></html>"); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse * response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub } }
9. Create html page in WebContent folder
index.html
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Java Tutorials Corner - Servlet - Request Dispatcher forward</title> </head> <body> <h1>Java Tutorials Corner - Servlet - Request Dispatcher forward</h1> <br /> <b>Request Dispatcher to Page</b> </body> </html>
9. Now save and Run the servlet, Run As –> Run on Server – Select your web Server to run the servlet. ( see How to configure tomcat in eclipse)
http://www.javatutorialcorner.com/2014/03/how-to-configure-tomcat-in-eclipse.html
10.call the URL which is mapped in web.xml.
http://localhost:8080/ServletExample/RequestDispatcherForward?target=servlet
Output
target=servlet

target=page
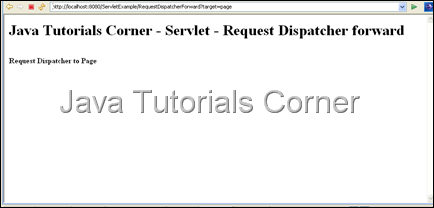
0 comments:
Post a Comment