In this tutorial we are going to see how to create web service end point and client in document style SAOP binding . Follow the steps given below to create SOAP web service.
1. Open Eclipse, Select File –> New --> Java Project
2. Create Java Project called WebService
3. Create package com.javatutorialscorner.jaxws.helloworld under WebService
4. Create Interface HelloWorld under package com.javatutorialscorner.jaxws.helloworld
HelloWorld.java
5. Create End Point Implementation class HelloWorldImpl under package com.javatutorialscorner.jaxws.helloworld
HelloWorldImpl.java
6.Create End point publisher class HelloWorldPublisher under package com.javatutorialscorner.jaxws.helloworld
HelloWorldPublisher .java
7.Now run the end point publisher class . Now your web service is published in the following URL
http://localhost:9080/helloworld
if you run the URL in web browser you can see the following screen
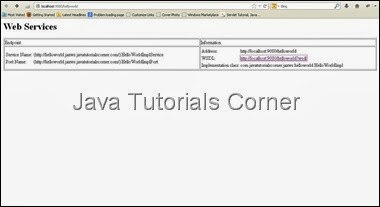
8.You can test the deployed web service by accessing the generated WSDL file via http://localhost:9080/helloworld?wsdl this URL
Generated WDSL file
9.You can access the web service in following way
1. Create New Java Project called WebServiceClient
2.Create package com.javatutorialscorner.jaxws.client under WebServiceClient
3. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client
HelloWorldClient.java
4. Run the client see the following output in console
1. Create New Java Project called WebServiceClient
2. Generate client stubs by running the following commads
or
3. You can see the following classes and interfaces generated in WebServiceClient project’s com.javatutorialscorner.jaxws.helloworld package
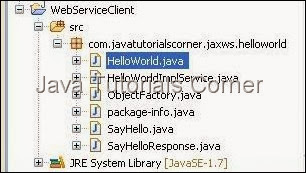
HelloWorld.java
HelloWorldImplService.java
ObjectFactory.java
package-info.java
SayHello.java
SayHelloResponse.java
4. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client to access the generated service
HelloWorldClient.java
5. Run the client see the following output in console
We will see wsgen tool in upcoming chapter.
1. Open Eclipse, Select File –> New --> Java Project
2. Create Java Project called WebService
3. Create package com.javatutorialscorner.jaxws.helloworld under WebService
4. Create Interface HelloWorld under package com.javatutorialscorner.jaxws.helloworld
HelloWorld.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.jws.WebMethod;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import javax.jws.soap.SOAPBinding.Style;
import javax.jws.soap.SOAPBinding.Use;
@WebService
@SOAPBinding(style = Style.DOCUMENT, use=Use.LITERAL)
public interface HelloWorld {
@WebMethod
public String sayHello(String message);
}
5. Create End Point Implementation class HelloWorldImpl under package com.javatutorialscorner.jaxws.helloworld
HelloWorldImpl.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.jws.WebService;
@WebService(endpointInterface = "com.javatutorialscorner.jaxws.helloworld.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
@Override
public String sayHello(String message) {
// TODO Auto-generated method stub
return "Java Tutorials Corner Says " + message;
}
}
6.Create End point publisher class HelloWorldPublisher under package com.javatutorialscorner.jaxws.helloworld
HelloWorldPublisher .java
package com.javatutorialscorner.jaxws.helloworld;
import javax.xml.ws.Endpoint;
public class HelloWorldPublisher {
public static void main(String[] args) {
// TODO Auto-generated method stub
Endpoint.publish("http://localhost:9080/helloworld",
new HelloWorldImpl());
}
}
7.Now run the end point publisher class . Now your web service is published in the following URL
http://localhost:9080/helloworld
if you run the URL in web browser you can see the following screen
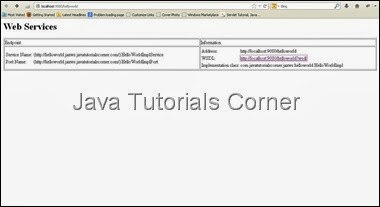
8.You can test the deployed web service by accessing the generated WSDL file via http://localhost:9080/helloworld?wsdl this URL
Generated WDSL file
<?xml version="1.0" encoding="UTF-8"?>
<!-- Published by JAX-WS RI at http://jax-ws.dev.java.net. RI's version is JAX-WS RI 2.2.4-b01. -->
<!-- Generated by JAX-WS RI at http://jax-ws.dev.java.net. RI's version is JAX-WS RI 2.2.4-b01. -->
<definitions xmlns:wsu="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd" xmlns:wsp="http://www.w3.org/ns/ws-policy" xmlns:wsp1_2="http://schemas.xmlsoap.org/ws/2004/09/policy" xmlns:wsam="http://www.w3.org/2007/05/addressing/metadata" xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:tns="http://helloworld.jaxws.javatutorialscorner.com/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns="http://schemas.xmlsoap.org/wsdl/" targetNamespace="http://helloworld.jaxws.javatutorialscorner.com/" name="HelloWorldImplService">
<types>
<xsd:schema>
<xsd:import namespace="http://helloworld.jaxws.javatutorialscorner.com/" schemaLocation="http://localhost:9080/helloworld?xsd=1"/>
</xsd:schema>
</types>
<message name="sayHello">
<part name="parameters" element="tns:sayHello"/>
</message>
<message name="sayHelloResponse">
<part name="parameters" element="tns:sayHelloResponse"/>
</message>
<portType name="HelloWorld">
<operation name="sayHello">
<input wsam:Action="http://helloworld.jaxws.javatutorialscorner.com/HelloWorld/sayHelloRequest" message="tns:sayHello"/>
<output wsam:Action="http://helloworld.jaxws.javatutorialscorner.com/HelloWorld/sayHelloResponse" message="tns:sayHelloResponse"/>
</operation>
</portType>
<binding name="HelloWorldImplPortBinding" type="tns:HelloWorld">
<soap:binding transport="http://schemas.xmlsoap.org/soap/http" style="document"/>
<operation name="sayHello">
<soap:operation soapAction=""/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
<service name="HelloWorldImplService">
<port name="HelloWorldImplPort" binding="tns:HelloWorldImplPortBinding">
<soap:address location="http://localhost:9080/helloworld"/>
</port>
</service>
</definitions>
9.You can access the web service in following way
- Access the web service using Java Client
- Access the web service using wsimport tool
- Access the web service using wsgen tool
1. Create New Java Project called WebServiceClient
2.Create package com.javatutorialscorner.jaxws.client under WebServiceClient
3. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client
HelloWorldClient.java
package com.javatutorialscorner.jaxws.client;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import com.javatutorialscorner.jaxws.helloworld.HelloWorld;
public class HelloWorldClient {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
URL url = new URL("http://localhost:9080/helloworld?wsdl");
QName qName = new QName(
"http://helloworld.jaxws.javatutorialscorner.com/",
"HelloWorldImplService");
Service service = Service.create(url, qName);
HelloWorld helloWorld = service.getPort(HelloWorld.class);
System.out.println("Web Service Message "
+ helloWorld.sayHello("Hello"));
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
4. Run the client see the following output in console
Access the web service using wsimport tool
Web Service Message Java Tutorials Corner Says Hello
1. Create New Java Project called WebServiceClient
2. Generate client stubs by running the following commads
cd %project_home%/src wsimport -s . http://localhost:9080/helloworld?wsdl
or
cd %project_home%/src wsimport -keep http://localhost:9080/helloworld?wsdl
3. You can see the following classes and interfaces generated in WebServiceClient project’s com.javatutorialscorner.jaxws.helloworld package
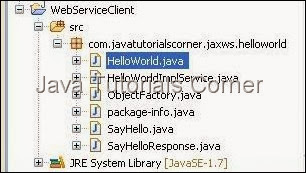
HelloWorld.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.xml.ws.RequestWrapper;
import javax.xml.ws.ResponseWrapper;
/**
* This class was generated by the JAXWS SI.
* JAX-WS RI 2.0_02-b08-fcs
* Generated source version: 2.0
*
*/
@WebService(name = "HelloWorld", targetNamespace = "http://helloworld.jaxws.javatutorialscorner.com/")
public interface HelloWorld {
/**
*
* @param arg0
* @return
* returns java.lang.String
*/
@WebMethod
@WebResult(targetNamespace = "")
@RequestWrapper(localName = "sayHello", targetNamespace = "http://helloworld.jaxws.javatutorialscorner.com/", className = "com.javatutorialscorner.jaxws.helloworld.SayHello")
@ResponseWrapper(localName = "sayHelloResponse", targetNamespace = "http://helloworld.jaxws.javatutorialscorner.com/", className = "com.javatutorialscorner.jaxws.helloworld.SayHelloResponse")
public String sayHello(
@WebParam(name = "arg0", targetNamespace = "")
String arg0);
}
HelloWorldImplService.java
package com.javatutorialscorner.jaxws.helloworld;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import javax.xml.ws.WebEndpoint;
import javax.xml.ws.WebServiceClient;
/**
* This class was generated by the JAXWS SI.
* JAX-WS RI 2.0_02-b08-fcs
* Generated source version: 2.0
*
*/
@WebServiceClient(name = "HelloWorldImplService", targetNamespace = "http://helloworld.jaxws.javatutorialscorner.com/", wsdlLocation = "http://localhost:9080/helloworld?wsdl")
public class HelloWorldImplService
extends Service
{
private final static URL HELLOWORLDIMPLSERVICE_WSDL_LOCATION;
static {
URL url = null;
try {
url = new URL("http://localhost:9080/helloworld?wsdl");
} catch (MalformedURLException e) {
e.printStackTrace();
}
HELLOWORLDIMPLSERVICE_WSDL_LOCATION = url;
}
public HelloWorldImplService(URL wsdlLocation, QName serviceName) {
super(wsdlLocation, serviceName);
}
public HelloWorldImplService() {
super(HELLOWORLDIMPLSERVICE_WSDL_LOCATION, new QName("http://helloworld.jaxws.javatutorialscorner.com/", "HelloWorldImplService"));
}
/**
*
* @return
* returns HelloWorld
*/
@WebEndpoint(name = "HelloWorldImplPort")
public HelloWorld getHelloWorldImplPort() {
return (HelloWorld)super.getPort(new QName("http://helloworld.jaxws.javatutorialscorner.com/", "HelloWorldImplPort"), HelloWorld.class);
}
}
ObjectFactory.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.javatutorialscorner.jaxws.helloworld package.
* <p>An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _SayHello_QNAME = new QName("http://helloworld.jaxws.javatutorialscorner.com/", "sayHello");
private final static QName _SayHelloResponse_QNAME = new QName("http://helloworld.jaxws.javatutorialscorner.com/", "sayHelloResponse");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.javatutorialscorner.jaxws.helloworld
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link SayHello }
*
*/
public SayHello createSayHello() {
return new SayHello();
}
/**
* Create an instance of {@link SayHelloResponse }
*
*/
public SayHelloResponse createSayHelloResponse() {
return new SayHelloResponse();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SayHello }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://helloworld.jaxws.javatutorialscorner.com/", name = "sayHello")
public JAXBElement<SayHello> createSayHello(SayHello value) {
return new JAXBElement<SayHello>(_SayHello_QNAME, SayHello.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SayHelloResponse }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://helloworld.jaxws.javatutorialscorner.com/", name = "sayHelloResponse")
public JAXBElement<SayHelloResponse> createSayHelloResponse(SayHelloResponse value) {
return new JAXBElement<SayHelloResponse>(_SayHelloResponse_QNAME, SayHelloResponse.class, null, value);
}
}
package-info.java
@javax.xml.bind.annotation.XmlSchema(namespace = "http://helloworld.jaxws.javatutorialscorner.com/")
package com.javatutorialscorner.jaxws.helloworld;
SayHello.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* <p>Java class for sayHello complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="sayHello">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="arg0" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "sayHello", propOrder = {
"arg0"
})
public class SayHello {
protected String arg0;
/**
* Gets the value of the arg0 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getArg0() {
return arg0;
}
/**
* Sets the value of the arg0 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setArg0(String value) {
this.arg0 = value;
}
}
SayHelloResponse.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* <p>Java class for sayHelloResponse complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="sayHelloResponse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="return" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "sayHelloResponse", propOrder = {
"_return"
})
public class SayHelloResponse {
@XmlElement(name = "return")
protected String _return;
/**
* Gets the value of the return property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReturn() {
return _return;
}
/**
* Sets the value of the return property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReturn(String value) {
this._return = value;
}
}
4. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client to access the generated service
HelloWorldClient.java
package com.javatutorialscorner.jaxws.client;
import com.javatutorialscorner.jaxws.helloworld.HelloWorld;
import com.javatutorialscorner.jaxws.helloworld.HelloWorldImplService;
public class HelloWorldClient {
public static void main(String[] args) {
HelloWorldImplService service = new HelloWorldImplService();
HelloWorld helloWorld = service.getHelloWorldImplPort();
System.out.println("Web Service message : "
+ helloWorld.sayHello("Hello"));
}
}
5. Run the client see the following output in console
Access the web service using wsgen tool
Web Service message : Java Tutorials Corner Says Hello
We will see wsgen tool in upcoming chapter.
0 comments:
Post a Comment