In this tutorial we are going to see how to create web service end point and client in RPC style SOAP binding . Follow the steps given below to create RPC style SOAP web service
1. Open Eclipse, Select File –> New --> Java Project
2. Create Java Project called WebService
3. Create package com.javatutorialscorner.jaxws.helloworld under WebService
4. Create Interface HelloWorld under package com.javatutorialscorner.jaxws.helloworld
HelloWorld.java
5. Create End Point Implementation class HelloWorldImpl under package com.javatutorialscorner.jaxws.helloworld
HelloWorldImpl.java
6.Create End point publisher class HelloWorldPublisher under package com.javatutorialscorner.jaxws.helloworld
HelloWorldPublisher .java
7.Now run the end point publisher class . Now your web service is published in the following URL
http://localhost:9080/helloworld
if you run the URL in web browser you can see the following screen
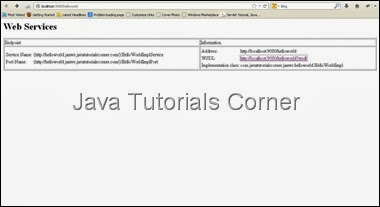
8.You can test the deployed web service by accessing the generated WSDL file via http://localhost:9080/helloworld?wsdl this URL
Generated WDSL file
9.You can access the web service in following way
1. Create New Java Project called WebServiceClient
2.Create package com.javatutorialscorner.jaxws.client under WebServiceClient
3. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client
HelloWorldClient.java
4. Run the client see the following output in console
Access the web service using wsimport tool
1. Create New Java Project called WebServiceClient
2. Generate client stubs by running the following commads
or
3. You can see the one classes and one interfaces generated in WebServiceClient project’s com.javatutorialscorner.jaxws.helloworld package.i.e
HelloWorldImplService.java
4. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client to access the generated service
HelloWorldClient.java
5. Run the client see the following output in console
1. Open Eclipse, Select File –> New --> Java Project
2. Create Java Project called WebService
3. Create package com.javatutorialscorner.jaxws.helloworld under WebService
4. Create Interface HelloWorld under package com.javatutorialscorner.jaxws.helloworld
HelloWorld.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.jws.WebMethod;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
import javax.jws.soap.SOAPBinding.Style;
@WebService
@SOAPBinding(style = Style.RPC)
public interface HelloWorld {
@WebMethod
public String sayHello(String message);
}
5. Create End Point Implementation class HelloWorldImpl under package com.javatutorialscorner.jaxws.helloworld
HelloWorldImpl.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.jws.WebService;
@WebService(endpointInterface = "com.javatutorialscorner.jaxws.helloworld.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
@Override
public String sayHello(String message) {
// TODO Auto-generated method stub
return "Java Tutorials Corner Says " + message;
}
}
6.Create End point publisher class HelloWorldPublisher under package com.javatutorialscorner.jaxws.helloworld
HelloWorldPublisher .java
package com.javatutorialscorner.jaxws.helloworld;
import javax.xml.ws.Endpoint;
public class HelloWorldPublisher {
public static void main(String[] args) {
// TODO Auto-generated method stub
Endpoint.publish("http://localhost:9080/helloworld",
new HelloWorldImpl());
}
}
7.Now run the end point publisher class . Now your web service is published in the following URL
http://localhost:9080/helloworld
if you run the URL in web browser you can see the following screen
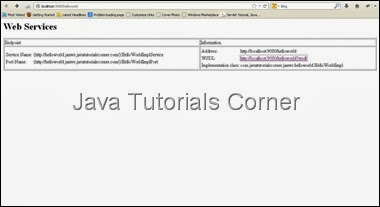
8.You can test the deployed web service by accessing the generated WSDL file via http://localhost:9080/helloworld?wsdl this URL
Generated WDSL file
<?xml version="1.0" encoding="UTF-8"?>
<!-- Published by JAX-WS RI at http://jax-ws.dev.java.net. RI's version is JAX-WS RI 2.2.4-b01. -->
<!-- Generated by JAX-WS RI at http://jax-ws.dev.java.net. RI's version is JAX-WS RI 2.2.4-b01. -->
<definitions xmlns:wsu="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd" xmlns:wsp="http://www.w3.org/ns/ws-policy" xmlns:wsp1_2="http://schemas.xmlsoap.org/ws/2004/09/policy" xmlns:wsam="http://www.w3.org/2007/05/addressing/metadata" xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:tns="http://helloworld.jaxws.javatutorialscorner.com/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns="http://schemas.xmlsoap.org/wsdl/" targetNamespace="http://helloworld.jaxws.javatutorialscorner.com/" name="HelloWorldImplService">
<types/>
<message name="sayHello">
<part name="arg0" type="xsd:string"/>
</message>
<message name="sayHelloResponse">
<part name="return" type="xsd:string"/>
</message>
<portType name="HelloWorld">
<operation name="sayHello">
<input wsam:Action="http://helloworld.jaxws.javatutorialscorner.com/HelloWorld/sayHelloRequest" message="tns:sayHello"/>
<output wsam:Action="http://helloworld.jaxws.javatutorialscorner.com/HelloWorld/sayHelloResponse" message="tns:sayHelloResponse"/>
</operation>
</portType>
<binding name="HelloWorldImplPortBinding" type="tns:HelloWorld">
<soap:binding transport="http://schemas.xmlsoap.org/soap/http" style="rpc"/>
<operation name="sayHello">
<soap:operation soapAction=""/>
<input>
<soap:body use="literal" namespace="http://helloworld.jaxws.javatutorialscorner.com/"/>
</input>
<output>
<soap:body use="literal" namespace="http://helloworld.jaxws.javatutorialscorner.com/"/>
</output>
</operation>
</binding>
<service name="HelloWorldImplService">
<port name="HelloWorldImplPort" binding="tns:HelloWorldImplPortBinding">
<soap:address location="http://localhost:9080/helloworld"/>
</port>
</service>
</definitions>
9.You can access the web service in following way
- Access the web service using Java Client
- Access the web service using wsimport tool
1. Create New Java Project called WebServiceClient
2.Create package com.javatutorialscorner.jaxws.client under WebServiceClient
3. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client
HelloWorldClient.java
package com.javatutorialscorner.jaxws.client;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import com.javatutorialscorner.jaxws.helloworld.HelloWorld;
public class HelloWorldClient {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
URL url = new URL("http://localhost:9080/helloworld?wsdl");
QName qName = new QName(
"http://helloworld.jaxws.javatutorialscorner.com/",
"HelloWorldImplService");
Service service = Service.create(url, qName);
HelloWorld helloWorld = service.getPort(HelloWorld.class);
System.out.println("Web Service Message "
+ helloWorld.sayHello("Hello"));
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
4. Run the client see the following output in console
HelloWorldClient.java
Web Service Message Java Tutorials Corner Says Hello
Access the web service using wsimport tool
1. Create New Java Project called WebServiceClient
2. Generate client stubs by running the following commads
cd %project_home%/src wsimport -s . http://localhost:9080/helloworld?wsdl
or
cd %project_home%/src wsimport -keep http://localhost:9080/helloworld?wsdl
3. You can see the one classes and one interfaces generated in WebServiceClient project’s com.javatutorialscorner.jaxws.helloworld package.i.e
- interface HelloWorld.java
- class HelloWorldImplService.java
package com.javatutorialscorner.jaxws.helloworld;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebResult;
import javax.jws.WebService;
import javax.jws.soap.SOAPBinding;
/**
* This class was generated by the JAXWS SI.
* JAX-WS RI 2.0_02-b08-fcs
* Generated source version: 2.0
*
*/
@WebService(name = "HelloWorld", targetNamespace = "http://helloworld.jaxws.javatutorialscorner.com/")
@SOAPBinding(style = SOAPBinding.Style.RPC)
public interface HelloWorld {
/**
*
* @param arg0
* @return
* returns java.lang.String
*/
@WebMethod
@WebResult(partName = "return")
public String sayHello(
@WebParam(name = "arg0", partName = "arg0")
String arg0);
}
HelloWorldImplService.java
package com.javatutorialscorner.jaxws.helloworld;
import java.net.MalformedURLException;
import java.net.URL;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import javax.xml.ws.WebEndpoint;
import javax.xml.ws.WebServiceClient;
/**
* This class was generated by the JAXWS SI.
* JAX-WS RI 2.0_02-b08-fcs
* Generated source version: 2.0
*
*/
@WebServiceClient(name = "HelloWorldImplService", targetNamespace = "http://helloworld.jaxws.javatutorialscorner.com/", wsdlLocation = "http://localhost:9080/helloworld?wsdl")
public class HelloWorldImplService
extends Service
{
private final static URL HELLOWORLDIMPLSERVICE_WSDL_LOCATION;
static {
URL url = null;
try {
url = new URL("http://localhost:9080/helloworld?wsdl");
} catch (MalformedURLException e) {
e.printStackTrace();
}
HELLOWORLDIMPLSERVICE_WSDL_LOCATION = url;
}
public HelloWorldImplService(URL wsdlLocation, QName serviceName) {
super(wsdlLocation, serviceName);
}
public HelloWorldImplService() {
super(HELLOWORLDIMPLSERVICE_WSDL_LOCATION, new QName("http://helloworld.jaxws.javatutorialscorner.com/", "HelloWorldImplService"));
}
/**
*
* @return
* returns HelloWorld
*/
@WebEndpoint(name = "HelloWorldImplPort")
public HelloWorld getHelloWorldImplPort() {
return (HelloWorld)super.getPort(new QName("http://helloworld.jaxws.javatutorialscorner.com/", "HelloWorldImplPort"), HelloWorld.class);
}
}
4. Create client class HelloWorldClient under com.javatutorialscorner.jaxws.client to access the generated service
HelloWorldClient.java
package com.javatutorialscorner.jaxws.client;
import com.javatutorialscorner.jaxws.helloworld.HelloWorld;
import com.javatutorialscorner.jaxws.helloworld.HelloWorldImplService;
public class HelloWorldClient {
public static void main(String[] args) {
HelloWorldImplService service = new HelloWorldImplService();
HelloWorld helloWorld = service.getHelloWorldImplPort();
System.out.println("Web Service message : "
+ helloWorld.sayHello("Hello"));
}
}
5. Run the client see the following output in console
Web Service message : Java Tutorials Corner Says Hello
0 comments:
Post a Comment