In this tutorial we are going to see how to download image file using RESTful web service
@Produces("image/png") annotation
1. Create new Dynamic web project by choosing File –> New –> Dynamic Web Project .
2. Create the Project called RESTful-WebService
3. Add the following jar into WEF-INF/lib folder
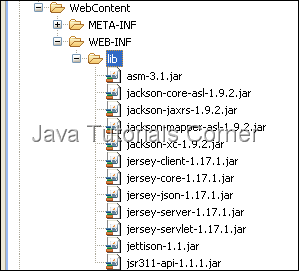
4. Create package called com.javatutorialscorner.jaxrs.downloadfile under RESTful-WebService
5. Create Java class DownloadImageFile under com.javatutorialscorner.jaxrs.downloadfile package
DownloadImageFile.java
package com.javatutorialscorner.jaxrs.downloadfile;
import java.io.File;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.ResponseBuilder;
@Path("/filedownload")
public class DownloadImageFile {
private static final String FILE_PATH = "C://jtc//java-tutorials.png";
@GET
@Path("/image-file")
@Produces("image/png")
public Response downloadFile() {
File file = new File(FILE_PATH);
ResponseBuilder responseBuilder = Response.ok((Object)file);
responseBuilder.header("Content-Disposition",
"attachment; filename=\"java-tutorials-corner.png\"");
return responseBuilder.build();
}
}
@Produces("image/png") used to set output response image file.
Content-Disposition in response header to tell user agent to pop up download box to download file
6.Configure Jersey Servlet Dispatcher
you need to configure REST as servlet in web.xml.
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<display-name>JAX-RS-Path</display-name>
<servlet>
<servlet-name>jersey-serlvet</servlet-name>
<servlet-class>
com.sun.jersey.spi.container.servlet.ServletContainer
</servlet-class>
<init-param>
<param-name>com.sun.jersey.config.property.packages</param-name>
<param-value>com.javatutorialscorner.jaxrs.downloadfile</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>jersey-serlvet</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
</web-app>
The servlet class available in jersey com.sun.jersey.spi.container.servlet.ServletContainer. The init param com.sun.jersey.config.property.package is used to define in which package jersey will look for the service classes.This package points to your resource class package. URL pattern is the part of base URL
Now you can run the service and access the service by calling the following URL
Output
http://localhost:8080/RESTful-WebService/rest/filedownload/image-file

0 comments:
Post a Comment