In previous tutorials we have seen simple Hello World RESTful Web service using JAX-RS.In this tutorial we will we see @PATH annotation used for URI in RESTful web service.follow the steps given below
Create new Dynamic Web Project and give project name as JAX-RS-Path
Copy all jar files into WEB-INF/lib folder.Select build path add jar in your build path.
Simple @Path Annotation
Create Class
Create package called com.javatutorialscorner.jaxrs.pathCreate following class inside package com.javatutorialscorner.jaxrs.path
RestPathService.java
package com.javatutorialscorner.jaxrs.path;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
@Path("/cricket")
public class RestPathService {
@GET
public Response getCaptain() {
return Response.status(200).entity("Captain : Dhoni").build();
}
@GET
@Path("/starplayer")
public Response getStarPlayer() {
return Response.status(200).entity("Cricket God : Sachin Tendulkar")
.build();
}
}
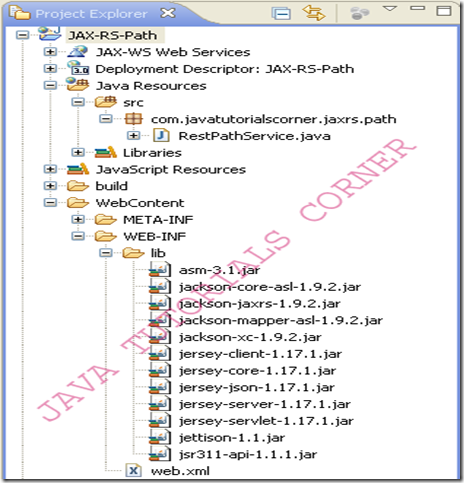
In this class getCaption() resource accessed by @PATH annotation declared for class with base URI and gerStarplayer() resource accessed by base URI + /cricket/starplayer. ‘starplayer’ is annotation for getStrarPlayer() method
Configure Jersey Servlet dispatcher
you need to register REST as servlet dispatcher in web.xml.Configure the following content in web.xmlweb.xml
JAX-RS-Path
jersey-serlvet
com.sun.jersey.spi.container.servlet.ServletContainer
com.sun.jersey.config.property.packages
com.javatutorialscorner.jaxrs.path
1
jersey-serlvet
/rest/*
http://your domain:port/Project Name/url pattern/pathOutput
http://localhost:8080/JAX-RS-Path/rest/cricket – access first method
http://localhost:8080/JAX-RS-Path/rest/cricket/starplayer – access second method
open browser enter the mention url you can see the following output
1. http://localhost:8080/JAX-RS-Path/rest/cricket
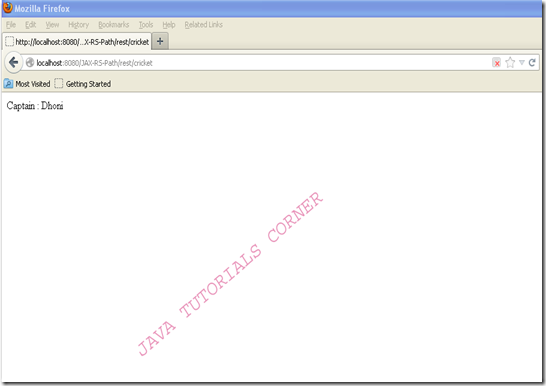
2.http://localhost:8080/JAX-RS-Path/rest/cricket/starplayer
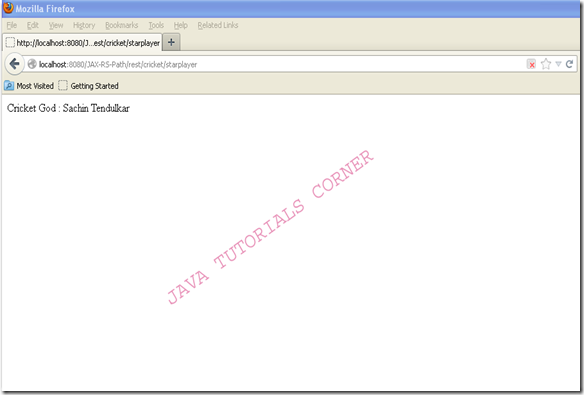
We used http GET method so we can able to call the resource by browser URL.We will see how to access these resources by using client code before that we will see other options available with @PATH annotation
URI with Path Param
RestPathParamService.javapackage com.javatutorialscorner.jaxrs.path;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.core.Response;
@Path("/shiningstar")
public class RestPathParamService {
@GET
@Path("{name}")
public Response getCricketer(@PathParam("name") String cricketer) {
return Response.status(200).entity("Young Shining Star : " + cricketer)
.build();
}
}
http://localhost:8080/JAX-RS-Path/rest/shiningstar/Kholi
here Kholi is parameter passed with URL.This parameter accessed by using @PathParam annotation
Output
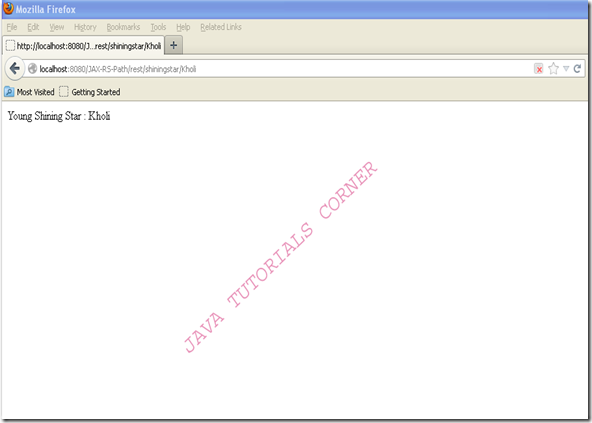
Regular Expression with Path
PathRegularExpression .javapackage com.javatutorialscorner.jaxrs.path;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.PathParam;
import javax.ws.rs.core.Response;
@Path("player")
public class PathRegularExpression {
@GET
@Path("/playerinfo/{crickername : [a-zA-Z]}")
public Response getPlayerName(@PathParam("crickername") String cricketer){
return Response.status(200).entity("Player Name : "+cricketer).build();
}
@GET
@Path("{age : \\d+}")
public Response getPlayerAge(@PathParam("age")String age){
return Response.status(200).entity("Player age : "+age).build();
}
}
Output:
http://localhost:8080/JAX-RS-Path/rest/player/D
accept single character String
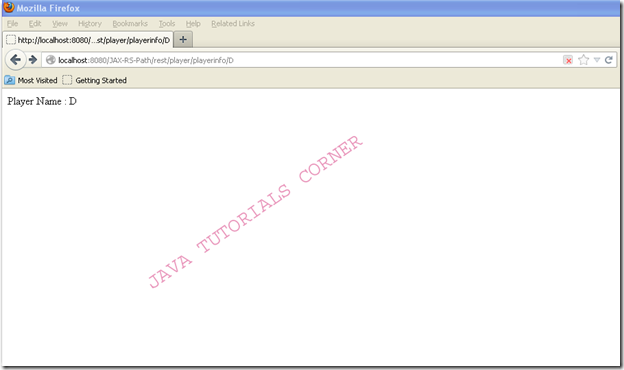
http://localhost:8080/JAX-RS-Path/rest/player/24
accept numerical digit
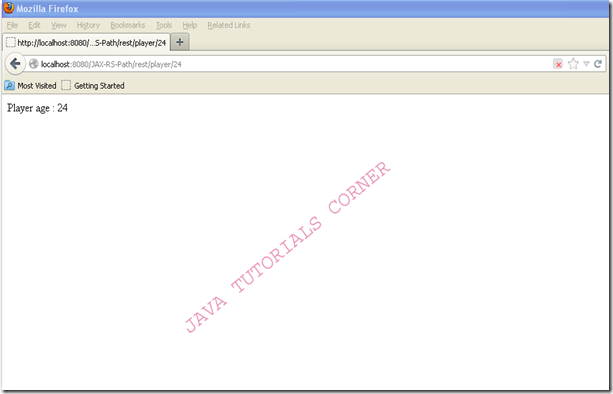
Client
RESTClient.javapackage com.javatutorialscorner.jaxrs.path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
public class RESTClient {
public static void main(String[] args) {
String baseURI = "http://localhost:8080/JAX-RS-Path";
ClientConfig config = new DefaultClientConfig();
Client client = Client.create(config);
WebResource service = client.resource(baseURI);
System.out.println("output for path param");
System.out.println(service.path("rest").path("shiningstar/Kholi")
.get(ClientResponse.class).getEntity(String.class));
System.out.println("output for smiple path");
System.out.println(service.path("rest").path("cricket")
.get(ClientResponse.class).getEntity(String.class));
System.out.println(service.path("rest").path("cricket/starplayer")
.get(ClientResponse.class).getEntity(String.class));
System.out.println("output for regular expression");
System.out.println(service.path("rest").path("player/playerinfo/D")
.get(ClientResponse.class).getEntity(String.class));
System.out.println(service.path("rest").path("player/24")
.get(ClientResponse.class).getEntity(String.class));
}
}
output for path param
Young Shining Star : Kholi
output for smiple path
Captain : Dhoni
Cricket God : Sachin Tendulkar
output for regular expression
Player Name : D
Player age : 24
0 comments:
Post a Comment