Create new Dynamic Web Project and give project name as RESTful_HelloWorld
Copy all jar files into WEB-INF/lib folder.Select build path add jar in your build path.
Create Class
Create package called com.javatutorialscorner.rest.wsCreate following class inside package com.javatutorialscorner.rest.ws
RestHelloWorld .java
package com.javatutorialscorner.rest.ws;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class RestHelloWorld {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello(){
return "Welcome to Java Tutorials Corner";
}
}
This class called by using base url + path and get resource using HTTP GET method its is specified by annotation @GET and it produce the Sting output as Plain Text output and @PATH define the class URI
now your project directory structure look like this
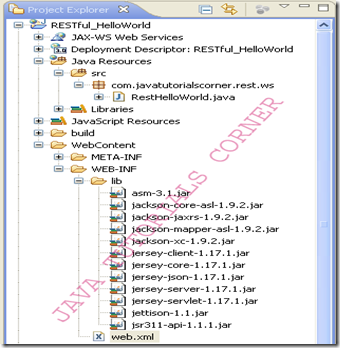
Configure Jersey Servlet dispatcher
you need to register REST as servlet dispatcher in web.xml.Configure the following content in web.xmlweb.xml
Restful_HelloWorld
jersey-serlvet
com.sun.jersey.spi.container.servlet.ServletContainer
com.sun.jersey.config.property.packages
com.javatutorialscorner.rest.ws
1
jersey-serlvet
/rest/*
This is servlet class available in jersey com.sun.jersey.spi.container.servlet.ServletContainer.The init param com.sun.jersey.config.property.packages is used to defines in which package jersey will look for the web service classes.This package point to your resource class package.URL pattern is part of your base URL.Now your web service is read to run.Your Web Service available at following url
http://localhost:8080/RESTful_HelloWorld/rest/hello
http://your domain:port/Project Name/url pattern/path
Output
open browser enter the mention url you can see the following output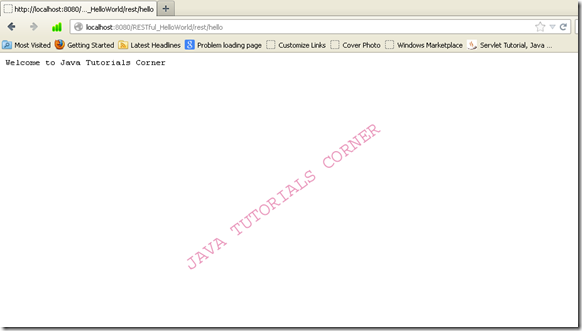
We can able to call GET method alone via browsers other methods of http should be called via client application
Create Client
you can create and run your client anywhere as per your wish in this example I created client inside same packageHelloWorldClient.java
package com.javatutorialscorner.rest.ws;
import javax.ws.rs.core.MediaType;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.WebResource;
import com.sun.jersey.api.client.config.ClientConfig;
import com.sun.jersey.api.client.config.DefaultClientConfig;
public class HelloWorldClient {
public static void main(String[] args) {
String baseURI = "http://localhost:8080/RESTful_HelloWorld";
ClientConfig config = new DefaultClientConfig();
Client client = Client.create(config);
WebResource service = client.resource(baseURI);
System.out.println(service.path("rest").path("hello")
.accept(MediaType.TEXT_PLAIN).get(String.class));
}
}
If you run this client you will get the following out in console
Welcome to Java Tutorials Corner
This comment has been removed by a blog administrator.
ReplyDelete